我拿到的開發(fā)板實際板載的MCU是GD32F310G8,QFN28pin封裝,基于ARM CORTEX M4內(nèi)核,主頻72MHz,芯片內(nèi)置64KB flash,8KB SRAM,兩路I2C外設(shè)。
本次試用目的是利用GD32F310的I2C0實現(xiàn)對HDC1080的初始化及讀取環(huán)境溫濕度,并利用UART口在電腦上顯示出來。
1、新建工程
①首先建立一個新的項目文件夾,并在此文件夾下建立對應(yīng)子文件夾,我建立的文件如下圖所示,這個依個人習慣會有不同:
② Document文件夾中存放對項目的說明文件readme.txt;將系統(tǒng)支持包中的GD32F3x0_Firmware_Library_V2.2.0/Firmware文件夾中的內(nèi)容復制到Libraries文件夾中;Listing/Output文件夾用于存放項目編譯時生成的目標文件及l(fā)ist文件,在MDK的魔術(shù)棒中進行設(shè)置路徑;users文件夾中存放main文件及我們自已寫的代碼;Project文件夾中存放MDK項目文件;
③打開MDK keil5,首先要先導入GD32F310G文件支持包。
④建立新的項目,選擇所使用的MCU,我們選擇GD32F330G8,如下圖所示
⑤新項目中建立如下文件組,并導入啟動文件和外設(shè)支持包。
⑥點擊魔術(shù)棒工具進行項目設(shè)置。在C/C++選項中添加include文件路徑;
⑦點擊Debug選項進行GDLink的設(shè)置,要選擇CMSIS_DAP Debugger,并將Reset and Run打勾。
⑧這樣整個項目的配置基本就結(jié)束了,可以在main.c文件中建立一個空循環(huán),編譯測試項目建立是否正確無誤。
2、硬件映射
硬件映射的目的是按目前的硬件連接建立.h文件,如下圖所示:
因此基于開發(fā)板I2C0采用的是PB6/PB7引腳,因為需要打印讀出的值,UART0使用的是PA9/PA10引腳。
3、編寫代碼
①UART0初始化和printf函數(shù)重定向:
void UART_TypeInit(void) { gpio_deinit(UART0_GPIO_PORT); usart_deinit(USART0); rcu_periph_clock_enable(UART0_GPIO_PORT_CLK); gpio_mode_set(UART0_GPIO_PORT,GPIO_MODE_AF,GPIO_PUPD_NONE,UART0_TX_PIN | UART0_RX_PIN); gpio_output_options_set(UART0_GPIO_PORT,GPIO_OTYPE_OD,GPIO_OSPEED_MAX,UART0_TX_PIN | UART0_RX_PIN); gpio_af_set(UART0_GPIO_PORT,GPIO_AF_1,UART0_TX_PIN | UART0_RX_PIN); rcu_periph_clock_enable(UART0_CLK); usart_baudrate_set(USART0,UART0_Baudrate); usart_parity_config(USART0,USART_PM_NONE); usart_word_length_set(USART0,USART_WL_8BIT); usart_stop_bit_set(USART0,USART_STB_1BIT); usart_enable(USART0); usart_transmit_config(USART0,USART_TRANSMIT_ENABLE); usart_receive_config(USART0,USART_RECEIVE_ENABLE); } /* retarget the C library printf function to the USART */ int fputc(int ch, FILE *f) { usart_data_transmit(USART0, (uint8_t)ch); while(RESET == usart_flag_get(USART0,USART_FLAG_TBE)); return ch; }
②I2C0外設(shè)初始化:
void I2C0_TypeInit(void) { gpio_deinit(I2C0_GPIO_PORT); i2c_deinit(I2C0); rcu_periph_clock_enable(I2C0_GPIO_PORT_CLK); gpio_mode_set(I2C0_GPIO_PORT,GPIO_MODE_AF,GPIO_PUPD_NONE,I2C0_SCL_PIN | I2C0_SDA_PIN); gpio_output_options_set(I2C0_GPIO_PORT,GPIO_OTYPE_OD,GPIO_OSPEED_MAX,I2C0_SCL_PIN | I2C0_SDA_PIN); gpio_af_set(I2C0_GPIO_PORT,GPIO_AF_1,I2C0_SCL_PIN | I2C0_SDA_PIN); rcu_periph_clock_enable(I2C0_CLK); i2c_clock_config(I2C0,I2C0_Frequence,I2C_DTCY_2); i2c_mode_addr_config(I2C0,I2C_I2CMODE_ENABLE,I2C_ADDFORMAT_7BITS,I2C0_OWN_Address); i2c_enable(I2C0); i2c_ack_config(I2C0,I2C_ACK_ENABLE); }
③讀寄存器操作:
void I2C0_Register_Read(uint8_t* B_buffer, uint8_t read_address,uint16_t number_of_byte) { /* wait until I2C bus is idle */ while(i2c_flag_get(I2C0, I2C_FLAG_I2CBSY)); if(2 == number_of_byte){ i2c_ackpos_config(I2C0,I2C_ACKPOS_NEXT); } /* send a start condition to I2C bus */ i2c_start_on_bus(I2C0); /* wait until SBSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_SBSEND)); /* send slave address to I2C bus */ i2c_master_addressing(I2C0, HDC1080_Addr, I2C_TRANSMITTER); /* wait until ADDSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND)); /* clear the ADDSEND bit */ i2c_flag_clear(I2C0, I2C_FLAG_ADDSEND); /* wait until the transmit data buffer is empty */ while(SET != i2c_flag_get( I2C0 , I2C_FLAG_TBE)); /* enable I2C0*/ i2c_enable(I2C0); /* send the EEPROM's internal address to write to */ i2c_data_transmit(I2C0, read_address); /* wait until BTC bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_BTC)); delay_1ms(20); /* send a start condition to I2C bus */ i2c_start_on_bus(I2C0); /* wait until SBSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_SBSEND)); /* send slave address to I2C bus */ i2c_master_addressing(I2C0, HDC1080_Addr, I2C_RECEIVER); if(number_of_byte < 3){ /* disable acknowledge */ i2c_ack_config(I2C0,I2C_ACK_DISABLE); } /* wait until ADDSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND)); /* clear the ADDSEND bit */ i2c_flag_clear(I2C0,I2C_FLAG_ADDSEND); if(1 == number_of_byte){ /* send a stop condition to I2C bus */ i2c_stop_on_bus(I2C0); } /* while there is data to be read */ while(number_of_byte){ if(3 == number_of_byte){ /* wait until BTC bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_BTC)); /* disable acknowledge */ i2c_ack_config(I2C0,I2C_ACK_DISABLE); } if(2 == number_of_byte){ /* wait until BTC bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_BTC)); /* send a stop condition to I2C bus */ i2c_stop_on_bus(I2C0); } delay_1ms(1); /* wait until the RBNE bit is set and clear it */ if(i2c_flag_get(I2C0, I2C_FLAG_RBNE)){ /* read a byte from the EEPROM */ *B_buffer = i2c_data_receive(I2C0); /* point to the next location where the byte read will be saved */ B_buffer++; /* decrement the read bytes counter */ number_of_byte--; } } /* wait until the stop condition is finished */ while(I2C_CTL0(I2C0)&0x0200); /* enable acknowledge */ i2c_ack_config(I2C0, I2C_ACK_ENABLE); i2c_ackpos_config(I2C0, I2C_ACKPOS_CURRENT); }
④寫寄存器操作:
void I2C0_Byte_Write(uint8_t* P_buffer, uint8_t write_address) { uint8_t i; /* wait until I2C bus is idle */ while(i2c_flag_get(I2C0, I2C_FLAG_I2CBSY)); /* send a start condition to I2C bus */ i2c_start_on_bus(I2C0); /* wait until SBSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_SBSEND)); /* send slave address to I2C bus */ i2c_master_addressing(I2C0, HDC1080_Addr, I2C_TRANSMITTER); /* wait until ADDSEND bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_ADDSEND)); /* clear the ADDSEND bit */ i2c_flag_clear(I2C0,I2C_FLAG_ADDSEND); /* wait until the transmit data buffer is empty */ while(SET != i2c_flag_get(I2C0, I2C_FLAG_TBE)); /* send the EEPROM's internal address to write to : only one byte address */ i2c_data_transmit(I2C0, write_address); /* wait until BTC bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_BTC)); for(i=0;i<2;i++) { /* send the byte to be written */ i2c_data_transmit(I2C0, *(P_buffer+i)); /* wait until BTC bit is set */ while(!i2c_flag_get(I2C0, I2C_FLAG_TBE)); } /* send a stop condition to I2C bus */ i2c_stop_on_bus(I2C0); /* wait until the stop condition is finished */ while(I2C_CTL0(I2C0)&0x0200); }
⑤對HDC1080進行初始化并讀取其ID:
void HDC1080_Init(void) { uint8_t IDBuffer[2]={0}; uint8_t InitSetup[2]={0x90,0x00}; uint32_t SID[3]={0},MID=0,DID=0; I2C0_Byte_Write(InitSetup,CONFIGURATION); delay_1ms(20); I2C0_Register_Read(IDBuffer,DEVICE_ID,2); DID = (((uint32_t)IDBuffer[0])<<8 | IDBuffer[1]); printf("The DeviceID is 0x%x\n\r",DID); I2C0_Register_Read(IDBuffer,MANUFACTURE_ID,2); MID = ((uint16_t) IDBuffer[0]<<8 | IDBuffer[1]); printf("The ManufactureID is 0x%x\n\r",MID); I2C0_Register_Read(IDBuffer,FIRST_SID,2); SID[0] = ((uint16_t) IDBuffer[0]<<8 | IDBuffer[1]); I2C0_Register_Read(IDBuffer,MID_SID,2); SID[1] = ((uint16_t) IDBuffer[0]<<8 | IDBuffer[1]); I2C0_Register_Read(IDBuffer,LAST_SID,2); SID[2] = ((uint16_t) IDBuffer[0]<<8 | IDBuffer[1]); printf("The First bytes of the serial ID of the part is 0x%x\n\r",SID[0]); printf("The MID bytes of the serial ID of the part is 0x%x\n\r",SID[1]); printf("The Last bytes of the serial ID of the part is 0x%x\n\r",SID[2]); printf("\n\r"); }
⑥讀取溫濕度值并轉(zhuǎn)換成十進制顯示:
void readSensor(void) { //holds 2 bytes of data from I2C Line uint8_t Buffer_Byte[2]; //holds the total contents of the temp register uint16_t temp,hum; //holds the total contents of the humidity register double temperature=0,humidity=0 ; I2C0_Register_Read(Buffer_Byte,T_MEASUREMENT,2); temp = (((uint32_t)Buffer_Byte[0])<<8 | Buffer_Byte[1]); temperature = (double)(temp)/(65536)*165-40; printf("The temperature is %.2f\n\r",temperature); I2C0_Register_Read(Buffer_Byte,RH_MEASUREMENT,2); hum = (((uint32_t)Buffer_Byte[0])<<8 | Buffer_Byte[1]); humidity = (double)(hum)/(65536)*100; printf("The humidity is %.2f%%\n\r",humidity); }
⑦main函數(shù):
/*! \brief main function \param[in] none \param[out] none \retval none */ int main(void) { /* configure periphreal */ systick_Init(); LED_TypeInit(); // NVIC_config(); // KEY_EXTI_TypeInit(); UART_TypeInit(); I2C0_TypeInit(); printf("**********DEMO START**********\n\r"); printf("******************************\n\r"); HDC1080_Init(); while(1) { readSensor(); delay_1ms(1000); } }
4、編譯下載
①Build項目文件:
②沒有錯誤,直接下載到開發(fā)板中,可以打開串口看到讀出溫濕度每隔1S讀一次并打印出來,與環(huán)境溫度計比較取值還是比較準確的。
5、試用總結(jié)
GD32F310G8芯片采用4x4 QFN28封裝,結(jié)構(gòu)可以設(shè)計相當緊湊,軟件開發(fā)資源也是很豐富,雖然功能不算特別強大,但主流的外設(shè)基本都有囊括,在目前國產(chǎn)替代的大環(huán)境之下,應(yīng)用前景還是相當廣泛的。
-
I2C
+關(guān)注
關(guān)注
28文章
1477瀏覽量
123057 -
uart
+關(guān)注
關(guān)注
22文章
1219瀏覽量
101120
發(fā)布評論請先 登錄
相關(guān)推薦
hdc1080讀取溫濕度值為0
請問這段基于msp430的hdc1080傳感器的驅(qū)動程序如何讀取溫濕度數(shù)值?我需要將溫濕度用oied屏顯示出來
MSP430FR2433無法讀取HDC1080
HDC1080溫濕度傳感器的使用步驟
基于GD32F310開發(fā)板的AD多通道交流采樣計算
利用GD32F310的I2C0實現(xiàn)對HDC1080的初始化及讀取環(huán)境溫濕度
在GD32F310開發(fā)板上移植FreeRTOS的步驟相關(guān)資料分享
HDC1080 HDC1080 具有溫度傳感器的低功耗、高精度數(shù)字濕度傳感器
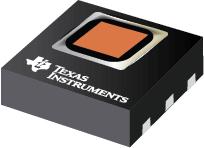
關(guān)于GD32F350R8的家庭環(huán)境智能控制系統(tǒng)的介紹和應(yīng)用
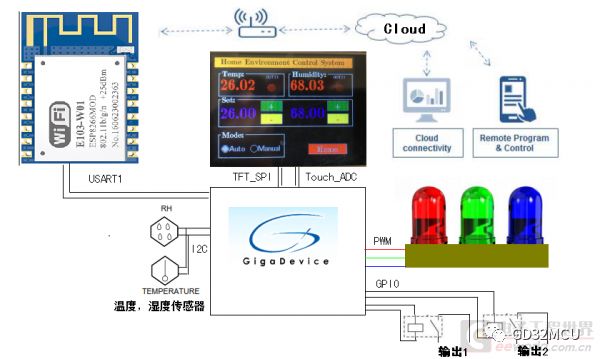
溫濕度記錄儀如何讀取,溫濕度記錄儀的操作介紹
HDC1080傳感器使用
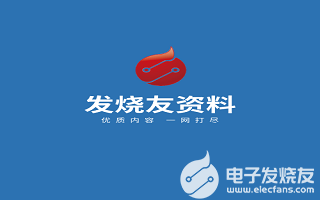
MSP432 P401R 單片機 讀取DHT11 串口發(fā)送溫濕度 OLED顯示溫濕度 溫濕度檢測
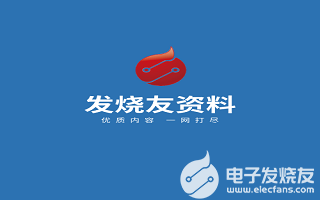
評論