這篇文章涉及到字符串與指針知識點的相關(guān)練習。浮點數(shù)與字符串互轉(zhuǎn)、字符串拷貝、字符串比較、指針交換變量、指針優(yōu)先級、數(shù)據(jù)類型強制轉(zhuǎn)換、內(nèi)存拷貝函數(shù)等。
1. 浮點數(shù)轉(zhuǎn)字符串
將字符串轉(zhuǎn)整數(shù)、整數(shù)轉(zhuǎn)字符串、浮點數(shù)轉(zhuǎn)字符串、字符串轉(zhuǎn)浮點數(shù) 封裝為函數(shù)。
浮點數(shù)轉(zhuǎn)字符串:
#include
#include //字符串處理
void float_to_string(char str[],float data);
int main(void)
{
char buff[50];
float data=1234.5;
float_to_string(buff,data);
printf("data=%f,buff=%s\n",data,buff);
return 0;
}
/*
函數(shù)功能: 浮點數(shù)轉(zhuǎn)字符串
*/
void float_to_string(char str[],float data)
{
int buff[50];
int int_data1=data; //提取整數(shù)部分
int int_data2=(data-int_data1)*1000000; //提取小數(shù)部分
int i=0,j=0,k=0;
//轉(zhuǎn)整數(shù)部分
while(int_data1)
{
buff[i]=int_data1%10+'0';
int_data1=int_data1/10;
i++;
}
//倒序
for(j=0;j=0; j--)
{
if(str[j]=='0')str[j]='\0'; //添加結(jié)束符
else break;
}
}
;j++)>
2. 封裝字符串拼接函數(shù)
封裝字符串拼接函數(shù):
函數(shù)功能實現(xiàn)將a和b字符串拼接在一起。
比如: char a[100]=”123”; char b[]=”456”; 調(diào)用函數(shù)之后: a[]=”123456”
#include
#include //字符串處理
void my_strcat(char str1[],char str2[]);
int main(void)
{
char str1[50]="12345";
char str2[]="67890";
//strcat(str1,str2); //字符串拼接
my_strcat(str1,str2); //字符串拼接
printf("%s\n",str1);
return 0;
}
/*
函數(shù)功能:字符串拼接
*/
void my_strcat(char str1[],char str2[])
{
int i=0,len=0;
while(str1[len]!='\0')
{
len++;
}
while(str2[i]!='\0')
{
str1[len+i]=str2[i];
i++;
}
str1[len+i]='\0'; //結(jié)尾補上'\0'
}
3. 封裝字符串的拷貝函數(shù)
封裝字符串的拷貝函數(shù): 將a字符串拷貝到b字符串。
示例:
#include
#include //字符串處理
void my_strcpy(char str1[],char str2[]);
int main(void)
{
char str1[50]="12345";
char str2[]="67890";
//strcpy(str1,str2); //字符串拷貝
my_strcpy(str1,str2);
printf("%s\n",str1);
return 0;
}
/*
函數(shù)功能:字符串拼接
*/
void my_strcpy(char str1[],char str2[])
{
int i=0,len=0;
while(str2[i]!='\0')
{
str1[i]=str2[i]; //依次賦值
i++;
}
str1[i]='\0'; //補上結(jié)尾符號
}
4. 封裝字符串的比較函數(shù)
封裝字符串的比較函數(shù): 比較a字符串和b字符串是否相等。 通過返回值進行區(qū)分。
示例:
#include
#include //字符串處理
int my_strcmp(char str1[],char str2[]);
int main(void)
{
char str1[50]="中國";
char str2[]="中國";
int a;
//a=strcmp(str1,str2);
a=my_strcmp(str1,str2);
if(a==0)printf("字符串相等!\n");
else printf("字符串不相等!\n");
return 0;
}
/*
函數(shù)功能:字符串比較
*/
int my_strcmp(char str1[],char str2[])
{
int i=0;
//只要有一個數(shù)組沒結(jié)束就繼續(xù)
while(str1[i]!='\0'||str2[i]!='\0')
{
if(str1[i]!=str2[i])break;
i++;
}
if(str1[i]=='\0' && str2[i]=='\0')return 0; //相等
else return -1; //不相等
}
5. 指針特性
指針: 是C語言的靈魂。
指針: 可訪問計算機的底層----->硬件。
定義指針的語法: <數(shù)據(jù)類型> *<變量名稱>;
int *p;
指針的特性:
(1)指針本身沒有空間。int *p; //定義一個指針變量
(2)指針本身就是地址(專門保存地址)。int *p; p=? ?必須是地址類型。
int *p; //定義一個指針變量
int data=123;
//p=123; //錯誤賦值方式
p=&data; //正確的賦值方式
(3)取出指針指向地址的數(shù)據(jù)
int *p; //定義一個指針變量
int data=123;
p=&data; //正確的賦值方式
printf("%d\n",*p); //取出p指針指向空間的值
//*p=888;
(4)指針類型的變量都是占4個字節(jié)
#include
int main()
{
int *p1; //定義一個整型指針變量
char *p2;
float *p3;
double *p4;
printf("int=%d\n",sizeof(p1));
printf("char=%d\n",sizeof(p2));
printf("float=%d\n",sizeof(p3));
printf("double=%d\n",sizeof(p4));
return 0;
}
(5)指針支持自增和自減 ++ --
#include
int main()
{
char *p;
char str[]="1234567890";
p=str; //將數(shù)組地址賦值給指針p
//指針可以直接使用數(shù)組下標(指針可以當數(shù)組名使用)
printf("%c\n",p[0]);//1
printf("%c\n",p[1]);//2
//通過指針訪問數(shù)組的成員
printf("%c\n",*p); //1
p++; //指針自增
printf("%c\n",*p); //2
p--;
printf("%c\n",*p); //1
return 0;
}
(6) 不同類型的指針自增和自減的字節(jié)數(shù)
//指針類型自增和自減的字節(jié)數(shù)與本身數(shù)據(jù)類型有關(guān)。
#include
int main()
{
int data1[10];
char data2[10];
/*1. 給指針賦值合法的空間*/
int *p1=data1;
char *p2=data2;
printf("0x%X\n",p1);
p1++;
printf("0x%X\n",p1);
printf("0x%X\n",p2);
p2++;
printf("0x%X\n",p2);
return 0;
}
6. 通過指針交換兩個變量的值
#include
void func(int *a,int *b);
int main()
{
int a=100,b=200;
func(&a,&b);
printf("a=%d,b=%d\n",a,b); //200,100
return 0;
}
//通過指針交換兩個變量的值
void func(int *a,int *b)
{
int c;
c=*a; //取出100
*a=*b; //取出200賦值給a
*b=c;
}
7. 指針自增優(yōu)先級
#include
int main()
{
char buff[]="12345";
char *p=buff;
printf("%c\n",*p); //1
printf("%c\n",*p++); //1
printf("%c\n",*p++); //2
printf("%c\n",*p); //3
return 0;
}
8. 計算字符串的長度
#include
int my_strlen(const char *str);
int main()
{
printf("%d\n",my_strlen("1234567"));
return 0;
}
//加const為了防止不小心修改了數(shù)據(jù)
int my_strlen(const char *str)
{
//*str='1'; //錯誤,不能賦值
char *p=str; //保存地址
while(*p!='\0')p++;
return p-str; //得到字符串長度
}
9. 數(shù)據(jù)類型的強制轉(zhuǎn)換
數(shù)據(jù)類型的強制轉(zhuǎn)換 (欺騙編譯器)
char *p1=(char*)src;
char *p2=(char*)new;
10. 編寫一個內(nèi)存拷貝函數(shù)
功能: 可以將任何數(shù)據(jù)的類型進行相互賦值。
int a,b=100; float a,b=123.456; ………..
#include
void my_memcpy(void *new,void *src,int len);
int main()
{
//int data1=123,data2=456;
//my_memcpy(&data1,&data2,4);
//printf("data1=%d\n",data1);
int data1[100];
int data2[50]={12,34,5,6,78};
my_memcpy(data1,data2,sizeof(data2));
printf("%d\n",data1[0]);
printf("%d\n",data1[1]);
return 0;
}
/*
內(nèi)存拷貝
將src的數(shù)據(jù)拷貝到new,拷貝len
*/
void my_memcpy(void *new,void *src,int len)
{
char *p1=(char*)src;
char *p2=(char*)new;
int i;
//for(i=0; i;>
-
C語言
+關(guān)注
關(guān)注
180文章
7594瀏覽量
135857 -
字符串
+關(guān)注
關(guān)注
1文章
575瀏覽量
20468 -
指針
+關(guān)注
關(guān)注
1文章
478瀏覽量
70491
發(fā)布評論請先 登錄
相關(guān)推薦
C語言字符串轉(zhuǎn)數(shù)字實現(xiàn)方法
C語言的字符串處理函數(shù)
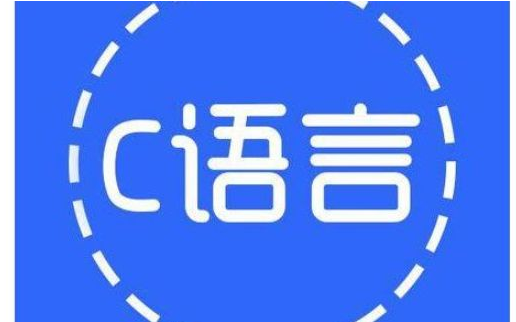
用指針實現(xiàn)字符串拷貝的程序和字符型指針變量與字符數(shù)組的區(qū)別說明
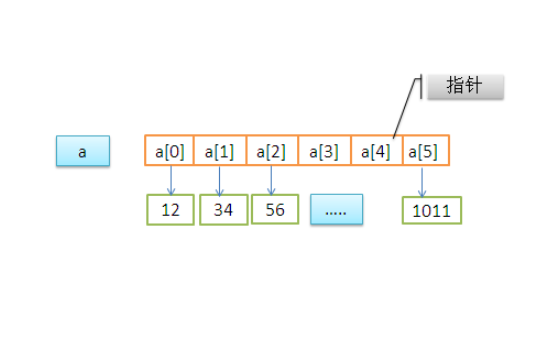
評論