背景
最近有一個需求是需要動態(tài)導出合同、訂單等信息,導出一個word文檔供客戶進行下載查看。
需要導出的word文件,主要可以分為兩種類型。
- 導出固定內容和圖片的word文檔
- 導出表格內容不固定的word文檔
經過對比工具,我實踐過兩種實現(xiàn)方式。第一種是FreeMarker模板來進行填充;第二種就是文中介紹的POI-TL。
這里我推薦使用POI-TL 。
介紹
POI-TL是word模板引擎,基于Apache POI,提供更友好的API。
目前最新的版本是1.12.X,POI對應版本是5.2.2。
這里需要注意的是POI和POI-TL有一個對應的關系。
準備工作
我使用的POI-TL版本是1.10.0
< dependency >
< groupId >com.deepoove< /groupId >
< artifactId >poi-tl< /artifactId >
< version >1.10.0< /version >
< /dependency >
< dependency >
< groupId >org.apache.poi< /groupId >
< artifactId >poi< /artifactId >
< version >4.1.2< /version >
< /dependency >
< dependency >
< groupId >org.apache.poi< /groupId >
< artifactId >poi-ooxml< /artifactId >
< version >4.1.2< /version >
< /dependency >
< dependency >
< groupId >org.apache.poi< /groupId >
< artifactId >poi-ooxml-schemas< /artifactId >
< version >4.1.2< /version >
< /dependency >
< dependency >
< groupId >commons-io< /groupId >
< artifactId >commons-io< /artifactId >
< version >2.7< /version >
< /dependency >
快速開始
流程:制作模板->提供數據->渲染模板->下載word
注意:需要填充的數據需要使用{{}}來表示。
1. 導出固定內容和圖片的word文檔
準備模板
模板保存為docx格式,存放在resource目錄下
提供數據
private Map< String, Object > assertMap() {
Map< String, Object > params = new HashMap< >();
params.put("name", "努力的螞蟻");
params.put("age", "18");
params.put("image", Pictures.ofUrl("http://deepoove.com/images/icecream.png").size(100, 100).create());
return params;
}
工具方法
/**
* 將項目中的模板文件拷貝到根目錄下
* @return
*/
private String copyTempFile(String templeFilePath) {
InputStream inputStream = getClass().getClassLoader().getResourceAsStream(templeFilePath);
String tempFileName = System.getProperty("user.home") + "/" + "1.docx";
File tempFile = new File(tempFileName);
try {
FileUtils.copyInputStreamToFile(inputStream, tempFile);
} catch (IOException e) {
throw new RuntimeException(e);
}
return tempFile.getPath();
}
private void down(HttpServletResponse response, String filePath, String realFileName) {
String percentEncodedFileName = null;
try {
percentEncodedFileName = percentEncode(realFileName);
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
StringBuilder contentDispositionValue = new StringBuilder();
contentDispositionValue.append("attachment; filename=").append(percentEncodedFileName).append(";").append("filename*=").append("utf-8''").append(percentEncodedFileName);
response.addHeader("Access-Control-Allow-Origin", "*");
response.addHeader("Access-Control-Expose-Headers", "Content-Disposition,download-filename");
response.setHeader("Content-disposition", contentDispositionValue.toString());
response.setHeader("download-filename", percentEncodedFileName);
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(filePath));
// 輸出流
BufferedOutputStream bos = new BufferedOutputStream(response.getOutputStream());) {
byte[] buff = new byte[1024];
int len = 0;
while ((len = bis.read(buff)) > 0) {
bos.write(buff, 0, len);
}
} catch (Exception e) {
e.printStackTrace();
}
}
/**
* 百分號編碼工具方法
* @param s 需要百分號編碼的字符串
* @return 百分號編碼后的字符串
*/
public static String percentEncode(String s) throws UnsupportedEncodingException {
String encode = URLEncoder.encode(s, StandardCharsets.UTF_8.toString());
return encode.replaceAll("\\\\+", "%20");
}
編寫接口
@RequestMapping("genera")
public void genera(HttpServletResponse response) {
//1.組裝數據
Map< String, Object > params = assertMap();
//2.獲取根目錄,創(chuàng)建模板文件
String path = copyTempFile("word/1.docx");
String fileName = System.currentTimeMillis() + ".docx";
String tmpPath = "D:\\\" + fileName;
try {
//3.將模板文件寫入到根目錄
//4.編譯模板,渲染數據
XWPFTemplate template = XWPFTemplate.compile(path).render(params);
//5.寫入到指定目錄位置
FileOutputStream fos = new FileOutputStream(tmpPath);
template.write(fos);
fos.flush();
fos.close();
template.close();
//6.提供前端下載
down(response, tmpPath, fileName);
} catch (Exception e) {
e.printStackTrace();
} finally {
//7.刪除臨時文件
File file = new File(tmpPath);
file.delete();
File copyFile = new File(path);
copyFile.delete();
}
}
對于圖片的格式,POI-TL也提供了幾種方式來提供支撐。
測試
效果如下:
2. 導出表格內容不固定的word文檔
表格動態(tài)內容填充,POI-TL提供了3種方式。
- 表格行循環(huán)
- 表格列循環(huán)
- 動態(tài)表格。
第二種和第三種都可以實現(xiàn)表格填充,但我個人感覺第一種更方便一點,這里我只介紹【表格行循環(huán)】實現(xiàn)方式。
LoopRowTableRenderPolicy
是一個特定場景的插件,根據集合數據循環(huán)表格行。
注意:
- 模板中有兩個list,這兩個list需要置于循環(huán)行的上一行。
- 循環(huán)行設置要循環(huán)的標簽和內容,注意此時的標簽應該使用[]
準備模板
提供數據
學生實體類
public class Student {
private String name;
private String age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
}
學生word類
public class StudentTable {
private String title;
private List< Student > studentList;
private List< Student > studentList1;
public List< Student > getStudentList1() {
return studentList1;
}
public void setStudentList1(List< Student > studentList1) {
this.studentList1 = studentList1;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public List< Student > getStudentList() {
return studentList;
}
public void setStudentList(List< Student > studentList) {
this.studentList = studentList;
}
}
表格數據
private StudentTable assertData() {
StudentTable table = new StudentTable();
table.setTitle("我是標題");
List< Student > studentList = new ArrayList< >();
Student student = new Student();
student.setName("張三");
student.setAge("18");
studentList.add(student);
Student student1 = new Student();
student1.setName("李四");
student1.setAge("20");
studentList.add(student1);
Student student2 = new Student();
student2.setName("王五");
student2.setAge("21");
studentList.add(student2);
Student student3 = new Student();
student3.setName("馬六");
student3.setAge("19");
studentList.add(student3);
table.setStudentList(studentList);
table.setStudentList1(studentList);
return table;
}
編寫接口
@RequestMapping("dynamicTable")
public void dynamicTable(HttpServletResponse response) {
//1.組裝數據
StudentTable table = assertData();
//2.獲取根目錄,創(chuàng)建模板文件
String path = copyTempFile("word/2.docx");
//3.獲取臨時文件
String fileName = System.currentTimeMillis() + ".docx";
String tmpPath = "D:\\\" + fileName;
try {
//4.編譯模板,渲染數據
LoopRowTableRenderPolicy hackLoopTableRenderPolicy = new LoopRowTableRenderPolicy();
Configure config =
Configure.builder().bind("studentList", hackLoopTableRenderPolicy).bind("studentList1", hackLoopTableRenderPolicy).build();
XWPFTemplate template = XWPFTemplate.compile(path, config).render(table);
//5.寫入到指定目錄位置
FileOutputStream fos = new FileOutputStream(tmpPath);
template.write(fos);
fos.flush();
fos.close();
template.close();
//6.提供下載
down(response, tmpPath, fileName);
} catch (Exception e) {
e.printStackTrace();
} finally {
//7.刪除臨時文件
File file = new File(tmpPath);
file.delete();
File copyFile = new File(path);
copyFile.delete();
}
}
測試
效果如下:
-
POI
+關注
關注
0文章
8瀏覽量
6998 -
API接口
+關注
關注
1文章
82瀏覽量
10420 -
SpringBoot
+關注
關注
0文章
173瀏覽量
161
發(fā)布評論請先 登錄
相關推薦
如何實現(xiàn)圖片轉Word文檔
基于多種技術的Word設計文檔自動生成平臺
一種基于Word文檔的數字密寫設計與實現(xiàn)
《微機原理及應用》課程教程 (word文檔)
一種快速Word編程接口的設計與實現(xiàn)
一種快速Word編程接口的設計與實現(xiàn)
如何在Word文檔中嵌入PROTEL原理圖
word文檔如何解密
用于損壞的Microsoft Word文檔的數據恢復軟件
求一種SpringBoot定時任務動態(tài)管理通用解決方案
如何提取Word文檔表格保存到Excel
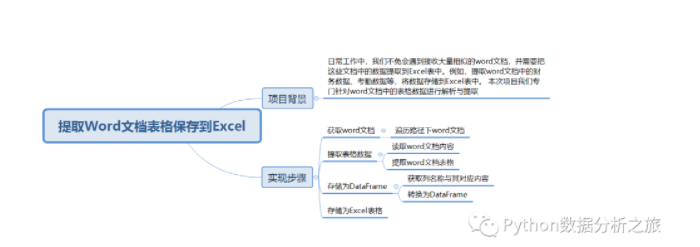
SpringBoot實現(xiàn)動態(tài)切換數據源

評論