Annotation
注解(Annotation),也叫元數據。一種代碼級別的說明。它是JDK1.5及以后版本引入的一個特性,與類、接口、枚舉是在同一個層次。它可以聲明在包、類、字段、方法、局部變量、方法參數等的前面,用來對這些元素進行說明,注釋。作用分類:
- 編寫文檔:通過代碼里標識的元數據生成文檔【生成文檔doc文檔】
- 代碼分析:通過代碼里標識的元數據對代碼進行分析【使用反射】
- 編譯檢查:通過代碼里標識的元數據讓編譯器能夠實現基本的編譯檢查【Override】
注解不會改變程序的語義,只是作為注解(標識)存在,我們可以通過反射機制編程實現對這些元數據(用來描述數據的數據)的訪問
分類
- 運行期注解 程序運行時才會被解析到的注解,一般通過反射機制來實現,很多框架中都會用到,經常會看到一個注解和一些簡單的配置來實現非常復雜的功能
- 編譯期注解 一般用來解析類型元數據,根據特定注解解析并生成代碼,或者生成一些描述性文件,比如properties、json等,比如為Pojo生成getter和setter方法
關鍵注解
@java.lang.annotation.Retention定義注解的有效時期
相關參數:RetentionPolicy.SOURCE: 編譯期生效,編譯器會丟棄,編譯后的class文件并不包含該注解 RetentionPolicy.CLASS: 注解會被保留在class文件中,但是運行期不會生效,被JVM忽略 RetentionPolicy.RUNTIME: 注解會被保留在class文件中,并且會在運行期生效,JVM會讀取
@Target定義注解作用對象,也就是注解是可以用在類、方法、參數還是其他等待
相關參數:ElementType.TYPE: 該注解只能運用到Class, Interface, enum上 ElementType.FIELD: 該注解只能運用到Field上 ElementType.METHOD: 該注解只能運用到方法上 ElementType.PARAMETER: 該注解只能作用在參數上 ElementType.CONSTRUCTOR: 該注解只能作用在構造方法上 ElementType.LOCAL_VARIABLE: 該注解作用在本地變量或catch語句 ElementType.ANNOTATION_TYPE: 該注解只能作用在注解上 ElementType.PACKAGE: 該注解只能用在包上
Java中常見的內置注解:
- @Override
- @Deprecated
- @SuppressWarnings
繼承關系
- @Inherited
如果某個注解上有@Inherited注解,當查找該類型的注解時,會先查找目標類型是否存在注解,如果有,直接返回;否則,繼續(xù)在父類上尋找注解, 停止的條件為在父類上找到該類型的注解或者父類為Object類型。
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@Inherited
public @interface ClassMapper {
}
下面的示例中,如果ClassMapper沒有@Inherited修飾,則返回null
Child.class.getAnnotation(ClassMapper.class);
@Slf4j
public class ExtendAnnotationTests {
@ClassMapper
public class Demo { }
public class Child extends Demo{ }
}
- 元注解 (注解上的注解)
我們知道,在Spring中,注解@Service與@Component都是用來標記類,交由Spring容器管理其對應的Bean,是結果是等效的。主要是Spring將注解和元注解進行了合并
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.ANNOTATION_TYPE)
public @interface Mapper {
}
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
@Mapper
public @interface ClassMapper {
}
通過下面的方法可以拿到元注解,從而進行其他擴展。
public class Tests {
@Test
public void test(){
ClassMapper classMapper = Demo.class.getAnnotation(ClassMapper.class);
log.info("classMapper: {}", classMapper);
Mapper mapper = classMapper.annotationType().getAnnotation(Mapper.class);
log.info("mapper: {}", mapper);
}
}
示例
示例主要針對@java.lang.annotation.Retention參數的三種情況,了解注解的生效時期:
RetentionPolicy.RUNTIME
該示例實現通過自定義注解@SystemProperty,實現為對象字段設置系統屬性
- 定義注解@SystemProperty
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
@Documented
public @interface SystemProperty {
String value();
}
- 定義對象工廠
主要作用是在運行時解析注解@SystemProperty,并實現系統屬性注入的邏輯。前面說到,注解的作用主要是標記,針對RetentionPolicy.RUNTIME類型的注解,一般是在運行時通過反射實現對注解標識的類、字段或方法等元素處理的過程。
ObjectFactory是一個對象生產工廠,這樣我們可以在運行期解析目標對象中的是否有@SystemProperty標識的字段,并對該字段進行值的設定,這是該注解設計的目的,但是具體實現需要我們根據需求來完成
@Slf4j
public class ObjectFactory {
// 省略 ...
public static < T > T getObject(Class< T > type, Object... args){
Constructor< T > constructor = findTypeConstructor(type, args);
T object = constructor.newInstance(args);
// 通過反射找到對象中@SystemProperty的字段,并根據其設置參數將系統屬性設定到該對象字段中
processFieldAnnotations(object, type, SystemProperty.class);
return object;
}
// 省略 ...
}
- 驗證
可以查看對象中被注解標識的屬性被設置上去了
@Slf4j
public class RuntimeAnnotationTests {
@Test
public void run(){
Demo demo = ObjectFactory.getObject(Demo.class);
log.info(" >> result: {}", demo.user);
}
@Data
public static class Demo{
@SystemProperty("user.name")
private String user;
}
}
RetentionPolicy.CLASS
該示例主要實現,編譯器判斷通過@FinalClass注解標記的類是否為final類型
- 定義注解
@Retention(RetentionPolicy.CLASS)
@Target(ElementType.TYPE)
@Documented
public @interface FinalClass {
}
- 編寫AbstractProcessor的實現
@SupportedAnnotationTypes({FinalClassProcessor.FINAL_CLASS})
@SupportedSourceVersion(SourceVersion.RELEASE_8)
@AutoService(Processor.class)
public class FinalClassProcessor extends AbstractProcessor {
public static final String FINAL_CLASS = "com.sucl.blog.jdk.annotation.compile.FinalClass";
@Override
public boolean process(Set< ? extends TypeElement > annotations, RoundEnvironment roundEnv) {
TypeElement annotationType = this.processingEnv.getElementUtils().getTypeElement(FINAL_CLASS);
if( annotationType != null ){
for (Element element : roundEnv.getElementsAnnotatedWith(annotationType)) {
if( element instanceof TypeElement ){
TypeElement typeElement = (TypeElement) element;
if( !typeElement.getModifiers().contains(Modifier.FINAL) ){
String message = String.format("類【%s】必須為final類型", typeElement);
this.processingEnv.getMessager().printMessage(Diagnostic.Kind.ERROR, message);
}
}
}
}
return true;
}
}
- 使FinalClassProcessor生效
- 基于google auto-service
3.1 添加依賴
< dependency >
< groupId >com.google.auto.service< /groupId >
< artifactId >auto-service< /artifactId >
< version >1.1.0< /version >
< /dependency >
3.2 在Processor通過注解@AutoService標識
@AutoService(Processor.class)
public class FinalClassProcessor extends AbstractProcessor{}
- 基于maven插件
< plugin >
< groupId >org.apache.maven.plugins< /groupId >
< artifactId >maven-compiler-plugin< /artifactId >
< configuration >
< annotationProcessors >
< annotationProcessor >
com.sucl.blog.jdk.annotation.compile.FinalClassProcessor
< /annotationProcessor >
< /annotationProcessors >
< /configuration >
< /plugin >
- 驗證
打包,在項目中引入該jar,定義一個類,類似下面這樣,當該類沒有final修飾時,通過maven install命令,可以看到控制臺打印自定義的錯誤信息
@FinalClass
public final class ProcessorFinder {}
注意
RetentionPolicy.CLASS的使用需要達打成jar包才行,不然無法再編譯時處理注解
RetentionPolicy.SOURCE
定義一個注解,通過打包后的結果觀察該注解的狀態(tài)
- 定義注解
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.TYPE)
@Documented
public @interface System {
}
- 定義測試類,并通過@System修飾
@System
public class SystemProvider {
}
- 打包,借助maven-source-plugin同時將源碼打包
< plugins >
< plugin >
< groupId >org.apache.maven.plugins< /groupId >
< artifactId >maven-source-plugin< /artifactId >
< version >3.2.1< /version >
< executions >
< execution >
< id >attach-sources< /id >
< goals >
< goal >jar< /goal >
< /goals >
< /execution >
< /executions >
< /plugin >
< /plugins >
- 在源碼包中,可以看到該注解仍然存在,但是class文件中卻沒有
在基于Spring Boot開發(fā)項目時,我們一般通過 @ConfigurationProperties 配合 spring-boot-configuration-processor ,可以實現在項目打包時 生成一個spring-configuration-metadata.json的配置描述文件,這樣在編寫application.yml配置時,就會得到配置提示,其實現方式就是基于 ConfigurationMetadataAnnotationProcessor,
結束語
注解本身沒有含義,主要作用是標記目標元素,后續(xù)拿到改標識的元數據,進行一系列的處理。注解的使用是非常廣泛的,各種框架中都使用頻繁,基于注解可以將很多抽象功能提取出來,通過簡單 的標識來實現各種復雜的功能
-
接口
+關注
關注
33文章
8447瀏覽量
150722 -
JAVA
+關注
關注
19文章
2952瀏覽量
104479 -
代碼
+關注
關注
30文章
4722瀏覽量
68231 -
編譯器
+關注
關注
1文章
1617瀏覽量
49015 -
元數據
+關注
關注
0文章
32瀏覽量
9119
發(fā)布評論請先 登錄
相關推薦
如何通過注解來優(yōu)化我們的Java代碼
HarmonyOS功能開發(fā)中常見問題
詳細介紹了Java泛型、注解、并發(fā)編程
HarmonyOS注解的使用方法分享
分析java注解基本概念
Spring Boot中常見的各類型注解的使用方式
注解定義Bean及開發(fā)
JAVA中注解是怎么做到的(上)
JAVA中注解是怎么做到的(下)
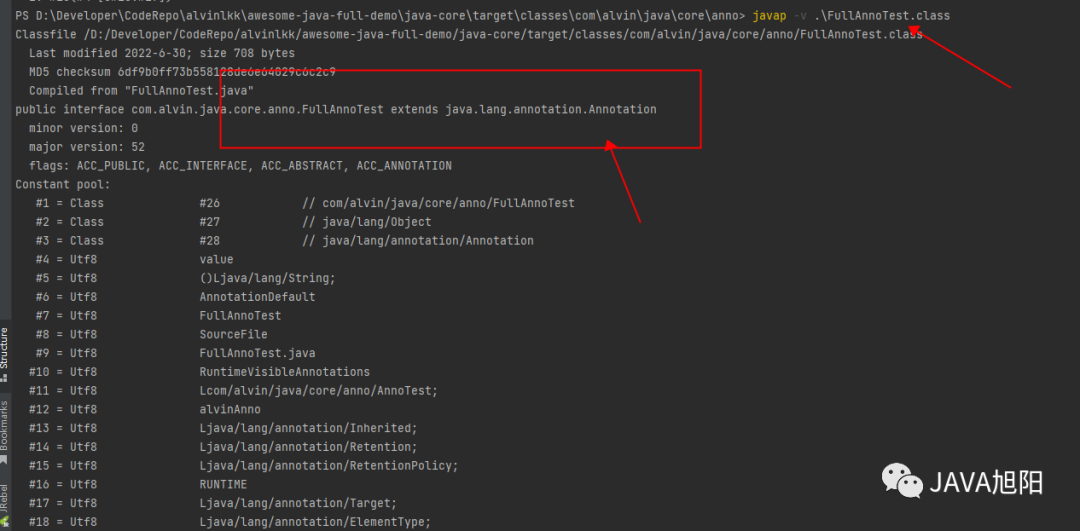
3分鐘純Java注解搭個管理系統
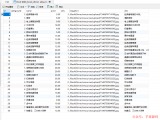
JDK中常見的Lamada表達式
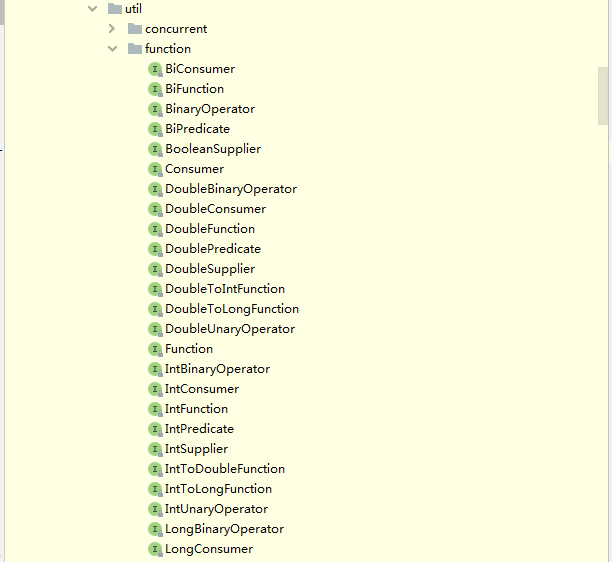
評論