今天我們講一下在 Java 中驗證碼的使用。
驗證碼生成
本效果是利用easy-captcha工具包實現(xiàn),首先需要添加相關(guān)依賴到pom.xml中,代碼如下:
< dependency >
< groupId >com.github.whvcse< /groupId >
< artifactId >easy-captcha< /artifactId >
< version >1.6.2< /version >
< /dependency >
驗證碼格式
easy-captcha驗證碼工具支持GIF、中文、算術(shù)等類型,分別通過下面幾個實例對象實現(xiàn):
- SpecCaptcha(PNG類型的靜態(tài)圖片驗證碼)
- GifCaptcha(Gif類型的圖片驗證碼)
- ChineseCaptcha(GIF類型中文圖片驗證碼)
- ArithmeticCaptcha(算術(shù)類型的圖片驗證碼)
字符類型分為以下幾種:
- TYPE_DEFAULT:數(shù)字和字母混合
- TYPEONLYNUMBER:純數(shù)字
- TYPEONLYCHAR:純字母
- TYPEONLYUPPER:純大寫字母
- TYPEONLYLOWER:純小寫字母
- TYPENUMAND_UPPER:數(shù)字和大寫字母混合
后端邏輯的實現(xiàn)
package com.yanx.controller;
import com.wf.captcha.SpecCaptcha;
import com.wf.captcha.base.Captcha;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.thymeleaf.util.StringUtils;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
@Controller
public class KapchaController {
@GetMapping("/kaptcha")
public void defaultKaptcha(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) throws IOException {
httpServletResponse.setHeader("Cache-Control","no-store");
httpServletResponse.setHeader("Pragma","no-cache");
httpServletResponse.setDateHeader("Expires",0);
httpServletResponse.setContentType("image/gif");
//三個參數(shù)分別為寬、高、位數(shù)
SpecCaptcha captcha=new SpecCaptcha(75,30,4);
//設(shè)置類型為數(shù)字和字母混合
captcha.setCharType(Captcha.TYPE_DEFAULT);
//設(shè)置字體
captcha.setCharType(Captcha.FONT_9);
//驗證碼存入session
httpServletRequest.getSession().setAttribute("verifyCode",captcha.text().toLowerCase());
//輸出圖片流
captcha.out(httpServletResponse.getOutputStream());
}
}
這里控制器新增了defaultKaptcha()方法,該方法所攔截處理的路徑為/kaptcha
前端邏輯的實現(xiàn)
在static目錄中新建kaptcha.html頁面,代碼如下:
< !DOCTYPE html >
< html lang="en" >
< head >
< meta charset="UTF-8" >
< title >驗證碼< /title >
< /head >
< body >
< img src="/kaptcha" onclick="this.src='/kaptcha?t=new Date()'" >
< /body >
< /html >
訪問后端驗證碼路徑/kaptcha,驗證碼為圖片形式。onclick方法為點擊該標(biāo)簽時可以動態(tài)切換顯示驗證碼。
啟動Spring Boot項目,打開瀏覽器輸入地址:
http://localhost:8080/kaptcha.html
效果如下:
驗證碼驗證
后端代碼
package com.yanx.controller;
import com.wf.captcha.SpecCaptcha;
import com.wf.captcha.base.Captcha;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.thymeleaf.util.StringUtils;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
@Controller
public class KapchaController {
@GetMapping("/kaptcha")
public void defaultKaptcha(HttpServletRequest httpServletRequest, HttpServletResponse httpServletResponse) throws IOException {
httpServletResponse.setHeader("Cache-Control","no-store");
httpServletResponse.setHeader("Pragma","no-cache");
httpServletResponse.setDateHeader("Expires",0);
httpServletResponse.setContentType("image/gif");
//三個參數(shù)分別為寬、高、位數(shù)
SpecCaptcha captcha=new SpecCaptcha(75,30,4);
//設(shè)置類型為數(shù)字和字母混合
captcha.setCharType(Captcha.TYPE_DEFAULT);
//設(shè)置字體
captcha.setCharType(Captcha.FONT_9);
//驗證碼存入session
httpServletRequest.getSession().setAttribute("verifyCode",captcha.text().toLowerCase());
//輸出圖片流
captcha.out(httpServletResponse.getOutputStream());
}
@GetMapping("/verify")
@ResponseBody
public String verify(@RequestParam("code") String code, HttpSession session){
if(StringUtils.isEmpty(code)){
return "驗證碼不能為空";
}
String kapchaCode = session.getAttribute("verifyCode")+"";
if(StringUtils.isEmpty(kapchaCode)||!code.toLowerCase().equals(kapchaCode)){
return "驗證碼輸入錯誤";
}
return "驗證成功";
}
}
前端代碼
< !DOCTYPE html >
< html lang="en" >
< head >
< meta charset="UTF-8" >
< title >驗證碼驗證< /title >
< /head >
< body >
< img src="/kaptcha" onclick="this.src='/kaptcha?d=new Date()'" >
< br >
< input type="text" maxlength="5" id="code" placeholder="請輸入驗證碼"/ >
< button id="verify" >驗證< /button >
< br/ >
< p id="verifyResult" >< /p >
< /body >
< script src="https://s3.pstatp.com/cdn/expire-1-M/jquery/3.3.1/jquery.min.js" >< /script >
< script type="text/javascript" >
$(function(){
//驗證按鈕點擊事件
$('#verify').click(function(){
var code=$('#code').val();
$.ajax({
type:'GET',//方法類型
url:'/verify?code='+code,
success:function(result){
$('#verifyResult').html(result);
},
error:function(){
alert('請求失敗');
},
});
});
});
< /script >
< /html >
效果
結(jié)束語
生成驗證碼功能還是比較常用的,所以記錄整理一下,方便以后回顧,如果有幫到你們的地方倍感榮幸,有路過的大佬還望不吝雅教!
-
JAVA
+關(guān)注
關(guān)注
19文章
2952瀏覽量
104477 -
瀏覽器
+關(guān)注
關(guān)注
1文章
1009瀏覽量
35226 -
代碼
+關(guān)注
關(guān)注
30文章
4722瀏覽量
68229 -
驗證碼
+關(guān)注
關(guān)注
2文章
20瀏覽量
4685
發(fā)布評論請先 登錄
相關(guān)推薦
10種意想不到的驗證碼風(fēng)格設(shè)計
java圖形驗證碼生成的設(shè)計實現(xiàn)
打碼平臺是如何高效的破解市面上各家驗證碼平臺的各種形式驗證碼的?
基于加密短信驗證碼的移動安全支付解決方案
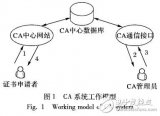
評論