列表去重是Python中一種常見的處理方式,任何編程場景都可能會遇到需要列表去重的情況。
列表去重的方式有很多,本文將一一講解他們,并進行性能的對比。
讓我們先制造一些簡單的數(shù)據(jù),生成0到99的100萬個隨機數(shù):
from random import randrange
DUPLICATES = [randrange(100) for _ in range(1000000)]
接下來嘗試這4種去重方式中最簡單直觀的方法:
1.新建一個數(shù)組,遍歷原數(shù)組,如果值不在新數(shù)組里便加入到新數(shù)組中。
# 第一種方式
def easy_way():
unique = []
for element in DUPLICATES:
if element not in unique:
unique.append(element)
return unique
進入ipython使用timeit計算其去重耗時:
%timeit easy_way()
# 1.16 s ± 137 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
平均耗時在1.16秒左右,但是在這個例子中我們使用了數(shù)組作為存儲對象,實際上如果我們改成集合存儲去重后的結(jié)果,性能會快不少:
def easy_way():
unique = set()
for element in DUPLICATES:
if element not in unique:
unique.add(element)
return unique
%timeit easy_way()
# 48.4 ms ± 11.6 ms per loop (mean ± std. dev. of 7 runs, 10 loops each)
平均耗時在48毫秒左右,改善明顯,這是因為集合和數(shù)組的內(nèi)在數(shù)據(jù)結(jié)構(gòu)完全不同,集合使用了哈希表,因此速度會比列表快許多,但缺點在于無序。
接下來看看第2種方式:
2.直接對數(shù)組進行集合轉(zhuǎn)化,然后再轉(zhuǎn)回數(shù)組:
# 第二種去重方式
def fast_way()
return list(set(DUPLICATES))
耗時:
%timeit fast_way()
# 14.2 ms ± 1.73 ms per loop (mean ± std. dev. of 7 runs, 100 loops each)
平均耗時14毫秒,這種去重方式是最快的,但正如前面所說,集合是無序的,將數(shù)組轉(zhuǎn)為集合后再轉(zhuǎn)為列表,就失去了原有列表的順序。
如果現(xiàn)在有保留原數(shù)組順序的需要,那么這個方式是不可取的,怎么辦呢?
3.保留原有數(shù)組順序的去重
使用dict.fromkeys()函數(shù),可以保留原有數(shù)組的順序并去重:
def save_order():
return list(dict.fromkeys(DUPLICATES))
當(dāng)然,它會比單純用集合進行去重的方式耗時稍微久一點:
%timeit save_order()
# 39.5 ms ± 8.66 ms per loop (mean ± std. dev. of 7 runs, 10 loops each)
平均耗時在39.5毫秒,我認(rèn)為這是可以接受的耗時,畢竟保留了原數(shù)組的順序。
但是,dict.fromkeys()僅在Python3.6及以上才支持。
如果你是Python3.6以下的版本,那么可能要考慮第四種方式了。
4. Python3.6以下的列表保留順序去重
在Python3.6以下,其實也存在fromkeys函數(shù),只不過它由collections提供:
from collections import OrderedDict
def save_order_below_py36():
return list(OrderedDict.fromkeys(DUPLICATES))
耗時:
%timeit save_order_below_py36()
# 71.8 ms ± 16.9 ms per loop (mean ± std. dev. of 7 runs, 10 loops each)
平均耗時在72毫秒左右,比 Python3.6 的內(nèi)置dict.fromkeys()慢一些,因為OrderedDict
是用純Python實現(xiàn)的。
-
數(shù)據(jù)
+關(guān)注
關(guān)注
8文章
6808瀏覽量
88743 -
存儲
+關(guān)注
關(guān)注
13文章
4226瀏覽量
85575 -
python
+關(guān)注
關(guān)注
55文章
4767瀏覽量
84375 -
數(shù)組
+關(guān)注
關(guān)注
1文章
412瀏覽量
25881
發(fā)布評論請先 登錄
相關(guān)推薦
python八種連接列表的方式
Python 編程常用的12種基礎(chǔ)知識匯總
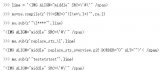
小猿圈python學(xué)習(xí)之Python列表list合并的4種方法
使用Python實現(xiàn)對excel文檔去重及求和的方法和代碼說明
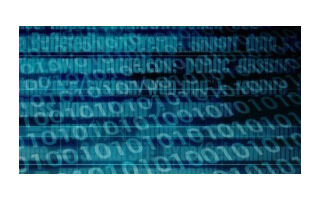
評論