流程圖:
一、簡單的交互
前端請求函數(shù)
firstGet(): Promise< AxiosResponse >{
return axios.get('http://192.168.211.1:8090/test/1');
}
getAaddB(a: number, b: number): Promise< AxiosResponse >{
return axios.get('http://192.168.211.1:8090/test/2', {
params: {
a: a,
b: b
}
})
}
這兩個函數(shù)是使用axios庫發(fā)起HTTP GET請求的函數(shù),用于與服務(wù)器進(jìn)行通信
- 服務(wù)器端點: http://192.168.211.1:8090/test/1 這是我本機的ip地址和springboot運行端口,使用在windows終端輸入ipconfig可查看
- 返回值: 該函數(shù)返回一個Promise,該Promise在請求成功時將包含AxiosResponse對象,其中包含了從服務(wù)器接收到的響應(yīng)信息。
后端controller
package com.example.demospring.controller;
import org.springframework.web.bind.annotation.*;
@RequestMapping("/test")
@RestController
public class test1 {
@GetMapping("/1")
public String test11(){
return "這是axios發(fā)送get請求從后端獲取的數(shù)據(jù)";
}
@GetMapping("/2")
public int AB(@RequestParam int a, @RequestParam int b){
return a + b;
}
}
test1()方法:
- 功能: 當(dāng)接收到GET請求 /test/1 時,該方法返回一個字符串 “這是axios發(fā)送get請求從后端獲取的數(shù)據(jù)”。
- 備注: 這是一個簡單的用于演示GET請求的方法,返回字符串?dāng)?shù)據(jù)。
二、axios與Spring Boot進(jìn)行前后端交互的實現(xiàn)
一、前后端交互配置
- Arkts目錄結(jié)構(gòu)
前端axios封裝一個簡單的網(wǎng)絡(luò)請求 在src/main/ets/network/AxiosRequest.ets里
import axios, { AxiosError, AxiosResponse, InternalAxiosRequestConfig } from '@ohos/axios' // 公共請求前綴 axios.defaults.baseURL = "http://192.168.211.1:8090" // 添加請求攔截器... // 添加響應(yīng)攔截器... // 導(dǎo)出 export default axios; export {AxiosResponse}
- 后端用于配置跨域資源共享(CORS)的設(shè)置 登錄后復(fù)制 @Override public void addCorsMappings(CorsRegistry registry) { registry.addMapping("/**") // 映射的路徑,這里是所有路徑 .allowedOrigins(" ") // 允許的來源,這里是所有來源,可以設(shè)置具體的域名或 IP 地址 .allowedMethods("PUT", "GET", "POST", "DELETE") // 允許的 HTTP 方法 .allowedHeaders(" ") // 允許的 HTTP 頭部 .allowCredentials(false) // 是否支持用戶憑據(jù),這里是不支持 .maxAge(300); // 預(yù)檢請求的有效期,單位秒 } @RequestMapping("/hello"):這個注解用于類級別,表示所有在這個控制器中的方法的URL映射的基本路徑 登錄后復(fù)制 @RestController @RequestMapping("/hello") public class SumUpController { ... }
二、不同請求的參數(shù)傳遞與后端接收返回代碼
1.get請求獲取數(shù)據(jù)
axios請求
export function get1(): Promise< AxiosResponse > {
return axios.get('/hello/get1');
}
后端controller
@GetMapping("/get1")
public String get1(){
return "這是你拿到的數(shù)據(jù)";
}
2.get請求傳遞多個參數(shù)
axios請求
export function get2(a: number, b: number): Promise< AxiosResponse > {
return axios.get('/hello/get2', {
//params字段包含了將要發(fā)送到后端的參數(shù)。
params: {
a: a,
b: b
}
});
}
后端controller
@GetMapping("/get2")
//使用@RequestParam注解從URL中獲取參數(shù)a和b。
public String get2(@RequestParam int a, @RequestParam int b){
return "你傳的兩個數(shù)是" + a + " " + b;
}
@RequestParam 注解允許你自定義請求參數(shù)的名稱,并提供其他選項,如設(shè)置默認(rèn)值或?qū)?shù)標(biāo)記為必需
3.get請求路徑參數(shù)
axios請求
export function get3(ps: number, pn: number): Promise< AxiosResponse > {
//注意要用``(反引號)
return axios.get(`/hello/get3/${pn}/${ps}`);
}
后端controller
@GetMapping("/get3/{pn}/{ps}")
public String get3(@PathVariable("ps") int ps, @PathVariable("pn") int pn){
return "你的查找要求是一頁" + ps + "條數(shù)據(jù)的第" + pn + "頁";
}
@PathVariable("id") 表示要從路徑中提取一個名為 “id” 的變量,并將其值綁定到 itemId 參數(shù)上。
4.get請求返回JSON數(shù)據(jù)
axios請求
//定義請求接收的數(shù)據(jù)類型
export interface ResponseData {
status: string;
message: string;
}
export function get4(): Promise< AxiosResponse< ResponseData > > {
return axios.get('/hello/get4');
}
Promise> 表示一個 Promise 對象,該對象最終解決為 Axios 發(fā)起的 HTTP 請求的響應(yīng),而該響應(yīng)的數(shù)據(jù)體應(yīng)該符合 ResponseData 類型的結(jié)構(gòu)。
后端controller
//@Data注解一個類時,Lombok會自動為該類生成常見的方法,如toString()、equals(),以及所有字段的getter和setter方法。
@Data
public static class ResponseData {
private String status;
private String message;
}
@GetMapping("/get4")
public ResponseData get4() {
ResponseData responseData = new ResponseData();
responseData.setStatus("success");
responseData.setMessage("這是一條成功的消息。");
return responseData;
}
5.post 使用對象作為請求參數(shù)
axios請求
export function post1(person: { name: string, age: number }): Promise< AxiosResponse > {
return axios.post(`/hello/post1`, person);
}
后端controller
@Data
public static class Person {
private String name;
private int age;
}
@PostMapping("/post1")
public String post1(@RequestBody Person person) {
return "你傳的姓名: " + person.getName() + " 年齡: " + person.getAge() + "。";
}
6.post 使用Map接收J(rèn)SON數(shù)據(jù)
axios請求
//JSON中,鍵和字符串值都應(yīng)該被雙引號包圍如
export function post2(data: any): Promise< AxiosResponse > {
return axios.post(`/hello/post2`, data);
}
后端controller
@PostMapping("/post2")
public String post2(@RequestBody Map< String, String > mp) {
AtomicReference< String > data = new AtomicReference< >("");
mp.forEach((k, v) - >{
data.set(data + k);
data.set(data + ": ");
data.set(data + v + ",");
});
return "你傳的鍵值對是: " + data;
}
7.put請求
axios請求
export function putExample(data: string): Promise< AxiosResponse > {
return axios.put('/hello/putExample', {data: data});
}
后端controller
@PutMapping("/putExample")
public String putExample(@RequestBody String data) {
return "這是PUT請求,傳入的數(shù)據(jù)是: " + data;
}
8.delete請求
axios請求
export function deleteExample(id: number): Promise< AxiosResponse > {
return axios.delete(`/hello/deleteExample/${id}`);
}
后端controller
@DeleteMapping("/deleteExample/{id}")
public String deleteExample(@PathVariable("id") int id) {
return "這是DELETE請求,要刪除的數(shù)據(jù)ID是: " + id;
}
三、調(diào)用傳參
import router from '@ohos.router'
import {get1, get2, get3, get4, post1, post2, putExample, deleteExample} from '../network/api/TestApi'
@Entry
@Component
struct Index {
@State get1: string = "";
@State get2: number = undefined;
@State get3: number = undefined;
@State get4: {status: string, message: string} = null;
@State post1: string = "";
@State post2: string = "";
@State put: string = "";
@State delete: string = "";
build() {
Column() {
Button("get1-get請求獲取數(shù)據(jù)")
.onClick(async () = >{
this.get1 = (await get1()).data;
})
Text(this.get1)
.fontSize(20)
Button("get2-傳遞多個參數(shù)")
.onClick(async () = >{
this.get2 = (await get2(1, 3)).data;
})
Text(`${this.get2!=undefined?this.get2:""}`)
.fontSize(20)
Button("get3-路徑參數(shù)")
.onClick(async () = >{
this.get3 = (await get3(3, 4)).data;
})
Text(`${this.get3!=undefined?this.get3:""}`)
.fontSize(20)
Button("get4-返回JSON數(shù)據(jù)")
.onClick(async () = >{
this.get4 = (await get4()).data;
})
Text(this.get4!=null ? JSON.stringify(this.get4) : "")
.fontSize(20)
Button("post1-使用對象作為請求參數(shù)")
.onClick(async () = >{
this.post1 = (await post1({name: "張三", age: 18})).data;
})
Text(this.post1)
.fontSize(20)
Button("post2-使用Map接收J(rèn)SON數(shù)據(jù)的POST請求示例")
.onClick(async () = >{
this.post2 = (await post2({id: "1", name: "李四", status: "call"})).data;
})
Text(this.post2)
.fontSize(20)
Button("put請求")
.onClick(async () = >{
this.put = (await putExample("put data")).data;
})
Text(this.put)
.fontSize(20)
Button("delete請求")
.onClick(async () = >{
this.delete = (await deleteExample(10)).data;
})
Text(this.delete)
.fontSize(20)
Button("對一個表單的增刪改查")
.margin(20)
.onClick(() = >{
router.pushUrl({
url: "pages/TalentTableTest"
})
})
}
.width('100%')
.height('100%')
.justifyContent(FlexAlign.Center)
}
}
以上就是鴻蒙開發(fā)的OpenHarmony;使用網(wǎng)絡(luò)組件axios與Spring Boot進(jìn)行前后端交互的技術(shù)解析,更多有關(guān)鴻蒙開發(fā)的學(xué)習(xí),可以去主頁查找,找我保存技術(shù)文檔。下面分享一張OpenHarmony學(xué)習(xí)路線圖 :
高清完整版曲線圖,可以找我保存 (附鴻蒙4.0&next版文檔)如下:
四、總結(jié)
一、請求參數(shù)錯誤的常見情況:
- 參數(shù)名稱不一致
- 參數(shù)類型(格式)不一致
- 缺少必須的請求參數(shù)
- 請求頭信息不符合要求
二、不同請求方式與參數(shù)傳遞方式的對應(yīng)關(guān)系:
- RESTful風(fēng)格的API通常使用路徑變量傳遞參數(shù)。在Spring框架中,可以使用@PathVariable注解來接收這些參數(shù)。
- URL中使用params傳遞參數(shù)時,通常使用@RequestParam注解來接收參數(shù)。
- 當(dāng)客戶端通過請求體傳遞JSON數(shù)據(jù)時,可以使用@RequestBody注解來接收。
- @ModelAttribute用于綁定方法參數(shù)或方法返回值到模型中,通常用于將請求參數(shù)與模型屬性進(jìn)行綁定。
審核編輯 黃宇
-
操作系統(tǒng)
+關(guān)注
關(guān)注
37文章
6684瀏覽量
123140 -
鴻蒙
+關(guān)注
關(guān)注
57文章
2302瀏覽量
42689 -
OpenHarmony
+關(guān)注
關(guān)注
25文章
3635瀏覽量
16061
發(fā)布評論請先 登錄
相關(guān)推薦
Spring Cloud Gateway網(wǎng)關(guān)框架
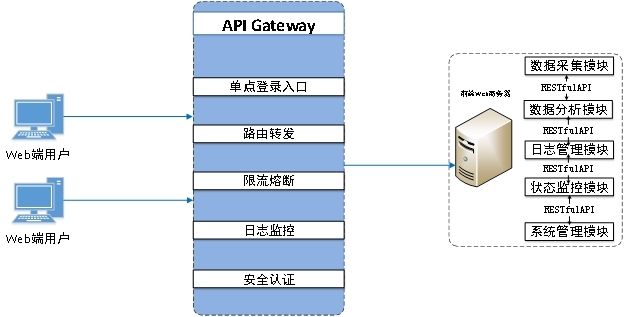
前后端數(shù)據(jù)傳輸約定探討
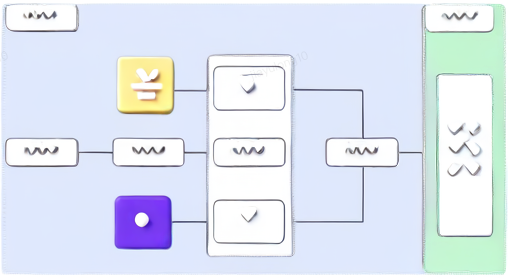
鴻蒙OS封裝【axios 網(wǎng)絡(luò)請求】(類似Android的Okhttp3)
鴻蒙開發(fā)實戰(zhàn):網(wǎng)絡(luò)請求庫【axios】
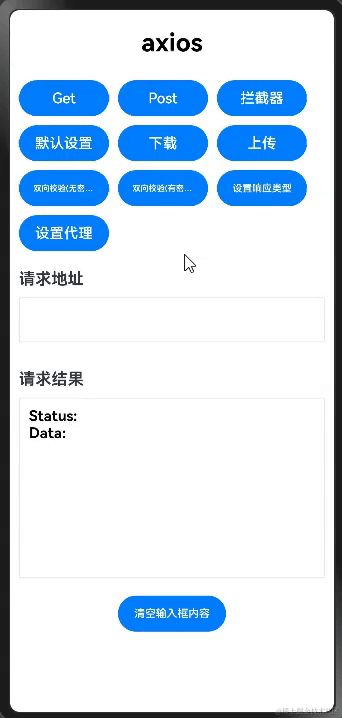
鴻蒙開發(fā)OpenHarmony組件復(fù)用案例
使用Spring Boot 3.2虛擬線程搭建靜態(tài)文件服務(wù)器
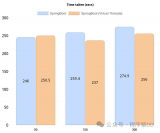
評論