要使用NumPy實(shí)現(xiàn)一個(gè)前饋神經(jīng)網(wǎng)絡(luò)(Feedforward Neural Network),我們需要從基礎(chǔ)開始構(gòu)建,包括初始化網(wǎng)絡(luò)參數(shù)、定義激活函數(shù)及其導(dǎo)數(shù)、實(shí)現(xiàn)前向傳播、計(jì)算損失函數(shù)、以及實(shí)現(xiàn)反向傳播算法來(lái)更新網(wǎng)絡(luò)權(quán)重和偏置。這里,我將詳細(xì)介紹一個(gè)包含單個(gè)隱藏層的前饋神經(jīng)網(wǎng)絡(luò)的實(shí)現(xiàn)。
一、引言
前饋神經(jīng)網(wǎng)絡(luò)是一種最基礎(chǔ)的神經(jīng)網(wǎng)絡(luò)結(jié)構(gòu),其中信息只向前傳播,不形成循環(huán)。它通常由輸入層、若干隱藏層(至少一層)和輸出層組成。在這個(gè)實(shí)現(xiàn)中,我們將構(gòu)建一個(gè)具有一個(gè)隱藏層的前饋神經(jīng)網(wǎng)絡(luò),用于解決二分類問題。
二、準(zhǔn)備工作
首先,我們需要導(dǎo)入NumPy庫(kù),并定義一些基本的函數(shù),如激活函數(shù)及其導(dǎo)數(shù)。
import numpy as np
def sigmoid(x):
"""Sigmoid激活函數(shù)"""
return 1 / (1 + np.exp(-x))
def sigmoid_derivative(x):
"""Sigmoid激活函數(shù)的導(dǎo)數(shù)"""
return x * (1 - x)
def mse_loss(y_true, y_pred):
"""均方誤差損失函數(shù)"""
return np.mean((y_true - y_pred) ** 2)
三、網(wǎng)絡(luò)結(jié)構(gòu)定義
接下來(lái),我們定義網(wǎng)絡(luò)的結(jié)構(gòu),包括輸入層、隱藏層和輸出層的節(jié)點(diǎn)數(shù)。
input_size = 3 # 輸入層節(jié)點(diǎn)數(shù)
hidden_size = 4 # 隱藏層節(jié)點(diǎn)數(shù)
output_size = 1 # 輸出層節(jié)點(diǎn)數(shù)
# 初始化權(quán)重和偏置
np.random.seed(0) # 為了可重復(fù)性設(shè)置隨機(jī)種子
weights_input_to_hidden = np.random.randn(input_size, hidden_size) * 0.01
bias_hidden = np.zeros((1, hidden_size))
weights_hidden_to_output = np.random.randn(hidden_size, output_size) * 0.01
bias_output = np.zeros((1, output_size))
四、前向傳播
前向傳播涉及將輸入數(shù)據(jù)通過網(wǎng)絡(luò)傳播到輸出層。
def forward_pass(X):
# 輸入層到隱藏層
hidden_layer_input = np.dot(X, weights_input_to_hidden) + bias_hidden
hidden_layer_output = sigmoid(hidden_layer_input)
# 隱藏層到輸出層
output_layer_input = np.dot(hidden_layer_output, weights_hidden_to_output) + bias_output
output_layer_output = sigmoid(output_layer_input)
return output_layer_output
五、反向傳播
反向傳播用于計(jì)算損失函數(shù)關(guān)于網(wǎng)絡(luò)參數(shù)的梯度,并據(jù)此更新這些參數(shù)。
def backward_pass(X, y_true, y_pred):
# 輸出層梯度
d_output = y_pred - y_true
d_output_wrt_output_input = sigmoid_derivative(y_pred)
d_hidden_to_output = d_output * d_output_wrt_output_input
# 更新輸出層權(quán)重和偏置
grad_weights_hidden_to_output = np.dot(hidden_layer_output.T, d_hidden_to_output)
grad_bias_output = np.sum(d_hidden_to_output, axis=0, keepdims=True)
# 隱藏層梯度
d_hidden = np.dot(d_hidden_to_output, weights_hidden_to_output.T)
d_hidden_wrt_hidden_input = sigmoid_derivative(hidden_layer_output)
d_input_to_hidden = d_hidden * d_hidden_wrt_hidden_input
# 更新輸入層到隱藏層的權(quán)重和偏置
grad_weights_input_to_hidden = np.dot(X.T, d_input_to_hidden)
grad_bias_hidden = np.sum(d_input_to_hidden, axis=0, keepdims=True)
return grad_weights_input_to_hidden, grad_bias_hidden, grad_weights_hidden_to_output, grad_bias_output
def update_parameters(def update_parameters(learning_rate, grad_weights_input_to_hidden, grad_bias_hidden, grad_weights_hidden_to_output, grad_bias_output):
"""
根據(jù)梯度更新網(wǎng)絡(luò)的權(quán)重和偏置。
Parameters:
- learning_rate: 浮點(diǎn)數(shù),學(xué)習(xí)率。
- grad_weights_input_to_hidden: 輸入層到隱藏層的權(quán)重梯度。
- grad_bias_hidden: 隱藏層的偏置梯度。
- grad_weights_hidden_to_output: 隱藏層到輸出層的權(quán)重梯度。
- grad_bias_output: 輸出層的偏置梯度。
"""
global weights_input_to_hidden, bias_hidden, weights_hidden_to_output, bias_output
weights_input_to_hidden -= learning_rate * grad_weights_input_to_hidden
bias_hidden -= learning_rate * grad_bias_hidden
weights_hidden_to_output -= learning_rate * grad_weights_hidden_to_output
bias_output -= learning_rate * grad_bias_output
# 示例數(shù)據(jù)
X = np.array([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6], [0.7, 0.8, 0.9]]) # 示例輸入
y_true = np.array([[0], [1], [0]]) # 示例真實(shí)輸出(二分類問題,使用0和1表示)
# 訓(xùn)練過程
epochs = 10000 # 訓(xùn)練輪次
learning_rate = 0.1 # 學(xué)習(xí)率
for epoch in range(epochs):
# 前向傳播
y_pred = forward_pass(X)
# 計(jì)算損失
loss = mse_loss(y_true, y_pred)
# 如果需要,可以在這里打印損失值以監(jiān)控訓(xùn)練過程
if epoch % 1000 == 0:
print(f'Epoch {epoch}, Loss: {loss}')
# 反向傳播
grad_weights_input_to_hidden, grad_bias_hidden, grad_weights_hidden_to_output, grad_bias_output = backward_pass(X, y_true, y_pred)
# 更新參數(shù)
update_parameters(learning_rate, grad_weights_input_to_hidden, grad_bias_hidden, grad_weights_hidden_to_output, grad_bias_output)
# 訓(xùn)練結(jié)束后,可以使用訓(xùn)練好的網(wǎng)絡(luò)進(jìn)行預(yù)測(cè)
# ...
六、評(píng)估與預(yù)測(cè)
在上面的代碼中,我們僅打印了訓(xùn)練過程中的損失值來(lái)監(jiān)控訓(xùn)練過程。在實(shí)際應(yīng)用中,你還需要在訓(xùn)練結(jié)束后對(duì)模型進(jìn)行評(píng)估,并使用它來(lái)對(duì)新數(shù)據(jù)進(jìn)行預(yù)測(cè)。評(píng)估通常涉及在一個(gè)與訓(xùn)練集獨(dú)立的測(cè)試集上計(jì)算模型的性能指標(biāo),如準(zhǔn)確率、召回率、F1分?jǐn)?shù)等。
七、擴(kuò)展與改進(jìn)
- 增加隱藏層 :可以通過增加更多的隱藏層來(lái)擴(kuò)展網(wǎng)絡(luò),構(gòu)建更深的神經(jīng)網(wǎng)絡(luò)。
- 使用不同的激活函數(shù) :除了Sigmoid外,還可以使用ReLU、Tanh等激活函數(shù),它們?cè)诓煌膽?yīng)用場(chǎng)景中可能表現(xiàn)更好。
- 引入正則化 :為了防止過擬合,可以在損失函數(shù)中加入正則化項(xiàng),如L1正則化、L2正則化或Dropout(雖然在這里我們手動(dòng)實(shí)現(xiàn)了網(wǎng)絡(luò),但NumPy本身不提供內(nèi)置的Dropout支持,通常需要使用其他庫(kù)如TensorFlow或PyTorch來(lái)實(shí)現(xiàn))。
- 優(yōu)化算法 :除了基本的梯度下降法外,還可以使用更高效的優(yōu)化算法,如Adam、RMSprop等。
- 批處理與數(shù)據(jù)增強(qiáng) :為了進(jìn)一步提高模型的泛化能力,可以使用批處理來(lái)加速訓(xùn)練過程,并使用數(shù)據(jù)增強(qiáng)技術(shù)來(lái)增加訓(xùn)練數(shù)據(jù)的多樣性。
八、結(jié)論
通過上面的介紹,我們了解了如何使用NumPy從頭開始實(shí)現(xiàn)一個(gè)具有單個(gè)隱藏層的前饋神經(jīng)網(wǎng)絡(luò)。雖然這個(gè)實(shí)現(xiàn)相對(duì)簡(jiǎn)單,但它為理解更復(fù)雜的深度學(xué)習(xí)模型和框架(如TensorFlow和PyTorch)奠定了基礎(chǔ)。在實(shí)際應(yīng)用中,我們通常會(huì)使用這些框架來(lái)構(gòu)建和訓(xùn)練神經(jīng)網(wǎng)絡(luò),因?yàn)樗鼈兲峁┝烁嗟墓δ芎蛢?yōu)化。然而,了解底層的實(shí)現(xiàn)原理對(duì)于深入理解深度學(xué)習(xí)仍然是非常重要的。
-
神經(jīng)網(wǎng)絡(luò)
+關(guān)注
關(guān)注
42文章
4733瀏覽量
100415 -
函數(shù)
+關(guān)注
關(guān)注
3文章
4277瀏覽量
62323
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
神經(jīng)網(wǎng)絡(luò)教程(李亞非)
【案例分享】基于BP算法的前饋神經(jīng)網(wǎng)絡(luò)
基于三層前饋BP神經(jīng)網(wǎng)絡(luò)的圖像壓縮算法解析
懲罰函數(shù)優(yōu)化的前饋神經(jīng)網(wǎng)絡(luò)盲多用戶檢測(cè)
基于遞歸神經(jīng)網(wǎng)絡(luò)和前饋神經(jīng)網(wǎng)絡(luò)的深度學(xué)習(xí)預(yù)測(cè)算法
基于Numpy實(shí)現(xiàn)同態(tài)加密神經(jīng)網(wǎng)絡(luò)
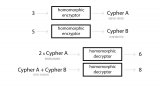
基于Numpy實(shí)現(xiàn)神經(jīng)網(wǎng)絡(luò):反向傳播
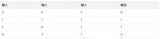
BP神經(jīng)網(wǎng)絡(luò)概述
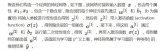
如何使用Numpy搭建神經(jīng)網(wǎng)絡(luò)
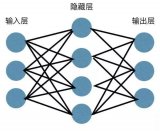
評(píng)論