1、程序簡(jiǎn)介
該程序是基于OpenHarmony的C++公共基礎(chǔ)類庫的讀寫鎖:rwlock。
本案例主要完成如下工作:
創(chuàng)建3個(gè)讀線程,每個(gè)讀線程循環(huán)5次,每次循環(huán)獲取讀鎖,將公共資源變量打印,睡眠1秒,然后釋放讀鎖,最后再睡眠1秒。
創(chuàng)建3個(gè)寫線程,每個(gè)寫線程循環(huán)5次,每次循環(huán)獲取寫鎖,將公共資源變量打印,睡眠1秒,然后釋放讀鎖,最后再睡眠1秒。
2、基礎(chǔ)知識(shí)
C++公共基礎(chǔ)類庫為標(biāo)準(zhǔn)系統(tǒng)提供了一些常用的C++開發(fā)工具類,包括:
文件、路徑、字符串相關(guān)操作的能力增強(qiáng)接口
讀寫鎖、信號(hào)量、定時(shí)器、線程增強(qiáng)及線程池等接口
安全數(shù)據(jù)容器、數(shù)據(jù)序列化等接口
各子系統(tǒng)的錯(cuò)誤碼相關(guān)定義
2.1、添加C++公共基礎(chǔ)類庫依賴
修改需調(diào)用模塊的BUILD.gn,在external_deps或deps中添加如下:
ohos_shared_library("xxxxx") { ... external_deps = [ ... # 動(dòng)態(tài)庫依賴(可選) "c_utils:utils", # 靜態(tài)庫依賴(可選) "c_utils:utilsbase", # Rust動(dòng)態(tài)庫依賴(可選) "c_utils:utils_rust", ] ...}
一般而言,我們只需要填寫"c_utils:utils"即可。
2.2、rwlock頭文件
C++公共基礎(chǔ)類庫的rwlock頭文件在://commonlibrary/c_utils/base/include/rwlock.h
可在源代碼中添加如下:
#include
OpenHarmony讀寫鎖分為如下三大類:
(1)RWLock類
OHOS::RWLock
(2)UniqueWriteGuard類
OHOS::UniqueWriteGuard
(3)UniqueReadGuard類
OHOS::UniqueReadGuard
2.3、OHOS::RWLock接口說明
2.3.1、RWLock
構(gòu)造函數(shù)。
RWLock() : RWLock(true);RWLock(bool writeFirst);
參數(shù)說明:
參數(shù)名稱 | 類型 | 參數(shù)說明 |
---|---|---|
writeFirst | bool | 是否優(yōu)先寫模式 |
2.3.2、~RWLock
析構(gòu)函數(shù)。
~RWLock();
2.3.3、LockRead
獲取讀鎖。
void LockRead();
2.3.4、UnLockRead
釋放讀鎖。
void UnLockRead();
2.3.5、LockWrite
獲取寫鎖。
void LockWrite();
2.3.6、UnLockWrite
獲取寫鎖。
void UnLockWrite();
2.4、OHOS::UniqueWriteGuard接口說明
2.4.1、UniqueWriteGuard
構(gòu)造函數(shù)。
UniqueWriteGuard(RWLockable &rwLockable)
參數(shù)說明:
參數(shù)名稱 | 類型 | 參數(shù)說明 |
---|---|---|
rwLockable | RWLockable | template |
2.4.2、~UniqueWriteGuard
析構(gòu)函數(shù)。
~UniqueWriteGuard();
2.5、OHOS::UniqueReadGuard接口說明
2.5.1、UniqueReadGuard
構(gòu)造函數(shù)。
UniqueReadGuard(RWLockable &rwLockable)
參數(shù)說明:
參數(shù)名稱 | 類型 | 參數(shù)說明 |
---|---|---|
rwLockable | RWLockable | template |
2.5.2、~UniqueReadGuard
析構(gòu)函數(shù)。
~UniqueReadGuard();
3、程序解析
3.1、創(chuàng)建編譯引導(dǎo)
在上一級(jí)目錄BUILD.gn文件添加一行編譯引導(dǎo)語句。
import("http://build/ohos.gni")
group("samples") { deps = [ "a25_utils_rwlock:utils_rwlock", # 添加該行 ]}
"a25_utils_rwlock:utils_rwlock",該行語句表示引入 參與編譯。
3.2、創(chuàng)建編譯項(xiàng)目
創(chuàng)建a25_utils_rwlock目錄,并添加如下文件:
a25_utils_rwlock├── utils_rwlock_sample.cpp # .cpp源代碼├──BUILD.gn#GN文件
3.3、創(chuàng)建BUILD.gn
編輯BUILD.gn文件。
import("http://build/ohos.gni")ohos_executable("utils_rwlock") { sources = [ "utils_rwlock_sample.cpp" ] include_dirs = [ "http://commonlibrary/c_utils/base/include", "http://commonlibrary/c_utils/base:utils", "http://third_party/googletest:gtest_main", "http://third_party/googletest/googletest/include" ] external_deps = [ "c_utils:utils" ] part_name = "product_rk3568" install_enable = true}
注意:
(1)BUILD.gn中所有的TAB鍵必須轉(zhuǎn)化為空格,否則會(huì)報(bào)錯(cuò)。如果自己不知道如何規(guī)范化,可以:
# 安裝gn工具sudo apt-get install ninja-buildsudo apt install generate-ninja# 規(guī)范化BUILD.gngn format BUILD.gn
3.4、創(chuàng)建源代碼
3.4.1、創(chuàng)建讀寫鎖和公共資源變量
static OHOS::RWLock m_rwlock(true);static int m_count = 0;
3.4.2、創(chuàng)建線程池并設(shè)置
int main(int argc, char **argv){ OHOS::ThreadPool threads("name_semaphore_threads"); int threads_start = 6; ...... threads.SetMaxTaskNum(128); threads.Start(threads_start); ......}
3.4.3、啟動(dòng)3個(gè)讀線程和3個(gè)寫線程
調(diào)用AddTask()添加子線程。
// 啟動(dòng)讀線程for (int i = 0; i < 3; i++) { str_name = "thread_read_" + to_string(i); auto task = std::bind(funcRead, str_name); threads.AddTask(task);}
// 啟動(dòng)寫線程for (int i = 0; i < 3; i++) { str_name = "thread_write_" + to_string(i); auto task = std::bind(funcWrite, str_name); threads.AddTask(task);}
3.4.4、編寫讀線程
讀線程完成如下步驟:
調(diào)用LockRead()獲取讀鎖;
如果獲取到讀鎖,打印公共資源變量,并睡眠1秒;
調(diào)用UnLockRead()釋放讀鎖;
睡眠1秒;
上述操作循環(huán)5次;
static void funcRead(const string& name){ cout << get_curtime() << ", " << name << ": start\n";
for (int i = 0; i < 5; i++) { cout << get_curtime() << ", " << name << ": LockRead wait..." << endl; m_rwlock.LockRead(); cout << get_curtime() << ", " << name << ": LockRead success and read count = " << m_count << " and sleep 1 sec" << endl; sleep(1); m_rwlock.UnLockRead(); cout << get_curtime() << ", " << name << ": UnLockRead" << endl; sleep(1); }}
3.4.5、編寫寫線程
寫線程完成如下步驟:
調(diào)用LockWrite()獲取寫鎖;
如果獲取到讀鎖,公共資源變量累加1,并睡眠1秒;
調(diào)用UnLockWrite()釋放寫鎖;
睡眠1秒;
上述操作循環(huán)5次;
static void funcWrite(const string& name){ cout << get_curtime() << ", " << name << ": start\n";
for (int i = 0; i < 5; i++) { cout << get_curtime() << ", " << name << ": LockWrite wait..." << endl; m_rwlock.LockWrite(); cout << get_curtime() << ", " << name << ": LockWrite success and write count++ and sleep 1 sec" << endl; m_count++; sleep(1); m_rwlock.UnLockWrite(); cout << get_curtime() << ", " << name << ": UnLockWrite" << endl; sleep(1); }}
4、編譯步驟
進(jìn)入OpenHarmony編譯環(huán)境,運(yùn)行命令:
hb build -f
5、運(yùn)行結(jié)果
# utils_rwlock2017-8-5 208, thread_read_0: start2017-8-5 20:7:8, thread_read_0: LockRead wait...2017-8-5 20:7:8, thread_read_0: LockRead success and read count = 0 and sleep 1 sec2017-8-5 20:7:8, thread_read_1: start2017-8-5 20:7:8, thread_read_1: LockRead wait...2017-8-5 20:7:8, thread_read_1: LockRead success and read count = 0 and sleep 1 sec2017-8-5 20:7:8, thread_read_2: start2017-8-5 20:7:8, thread_read_2: LockRead wait...2017-8-5 20:7:8, thread_read_2: LockRead success and read count = 0 and sleep 1 sec2017-8-5 20:7:8, thread_write_0: start2017-8-5 20:7:8, thread_write_0: LockWrite wait...2017-8-5 20:7:8, thread_write_1: start2017-8-5 20:7:8, thread_write_1: LockWrite wait...2017-8-5 20:7:9, thread_read_0: UnLockRead2017-8-5 20:7:9, thread_read_1: UnLockRead2017-8-5 20:7:9, thread_read_2: UnLockRead2017-8-5 20:7:9, thread_write_1: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:10, thread_read_0: LockRead wait...2017-8-5 20:7:10, thread_write_1: UnLockWrite2017-8-5 20:7:10, thread_write_0: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:10, thread_read_1: LockRead wait...2017-8-5 20:7:10, thread_read_2: LockRead wait...2017-8-5 20:7:112017-8-5 20:7:11, thread_write_0, thread_read_0: LockRead success and read count = : UnLockWrite2 and sleep 1 sec2017-8-5 20:7:11, thread_read_1: LockRead success and read count = 2 and sleep 1 sec2017-8-5 20:7:11, thread_write_1: LockWrite wait...2017-8-5 20:7:12, thread_write_0: LockWrite wait...2017-8-5 20:7:12, thread_read_1: UnLockRead2017-8-5 20:7:12, thread_read_0: UnLockRead2017-8-5 20:7:12, thread_write_0: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:13, thread_read_1: LockRead wait...2017-8-5 20:7:13, thread_read_0: LockRead wait...2017-8-5 20:7:13, thread_write_0: UnLockWrite2017-8-5 20:7:13, thread_write_1: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:14, thread_write_0: LockWrite wait...2017-8-5 20:7:14, thread_write_1: UnLockWrite2017-8-5 20:7:14, thread_write_0: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:15, thread_read_0: LockRead success and read count = 2017-8-5 20:7:15, thread_write_0: UnLockWrite2017-8-5 20:7:15, thread_write_1: LockWrite wait...2017-8-5 20:7:15, thread_read_1: LockRead success and read count = 5 and sleep 1 sec5 and sleep 1 sec2017-8-5 20:7:16, thread_write_0: LockWrite wait...2017-8-5 20:7:16, thread_read_1: UnLockRead2017-8-5 20:7:16, thread_read_0: UnLockRead2017-8-5 20:7:16, thread_write_1: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:17, thread_read_1: LockRead wait...2017-8-5 20:7:17, thread_read_0: LockRead wait...2017-8-5 20:7:17, thread_write_1: UnLockWrite2017-8-5 20:7:17, thread_write_0: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:18, thread_write_1: LockWrite wait...2017-8-5 20:7:18, thread_write_1: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:18,thread_write_0: UnLockWrite2017-8-5 20:7:192017-8-5 20:7:19, thread_write_0, thread_write_1: UnLockWrite: LockWrite wait...2017-8-5 20:7:19,
thread_read_1: LockRead success and read count = 8 and sleep 1 sec2017-8-5 20:7:19, thread_read_0: LockRead success and read count = 8 and sleep 1 sec2017-8-5 20:7:19, thread_read_2: LockRead success and read count = 8 and sleep 1 sec2017-8-5 20:7:20, thread_write_1: LockWrite wait...2017-8-5 20:7:20, thread_read_1: UnLockRead2017-8-5 20:7:20, thread_read_0: UnLockRead2017-8-5 20:7:20, thread_read_2: UnLockRead2017-8-5 20:7:20, thread_write_1: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:21, thread_read_1: LockRead wait...2017-8-5 20:7:21, thread_read_0: LockRead wait...2017-8-5 20:7:21, thread_read_2: LockRead wait...2017-8-5 20:7:21, thread_write_1: UnLockWrite2017-8-5 20:7:21, thread_write_0: LockWrite success and write count++ and sleep 1 sec2017-8-5 20:7:22, thread_write_0: UnLockWrite2017-8-5 20:7:22, thread_read_0: LockRead success and read count = 10 and sleep 1 sec2017-8-5 20:7:22, thread_read_1: LockRead success and read count = 10 and sleep 1 sec2017-8-5 20:7:22, thread_read_2: LockRead success and read count = 10 and sleep 1 sec2017-8-5 20:7:23, thread_read_0: UnLockRead2017-8-5 20:7:23, thread_read_1: UnLockRead2017-8-5 20:7:23, thread_read_2: UnLockRead2017-8-5 20:7:24, thread_read_2: LockRead wait...2017-8-5 20:7:24, thread_read_2: LockRead success and read count = 10 and sleep 1 sec2017-8-5 20:7:25, thread_read_2: UnLockRead2017-8-5 20:7:26, thread_read_2: LockRead wait...2017-8-5 20:7:26, thread_read_2: LockRead success and read count = 10 and sleep 1 sec2017-8-5 20:7:27, thread_read_2: UnLockRead#
-
程序
+關(guān)注
關(guān)注
116文章
3756瀏覽量
80751 -
系統(tǒng)
+關(guān)注
關(guān)注
1文章
1006瀏覽量
21291 -
OpenHarmony
+關(guān)注
關(guān)注
25文章
3635瀏覽量
16061
發(fā)布評(píng)論請(qǐng)先 登錄
相關(guān)推薦
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:ThreadPoll
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:Semaphore
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:rwlock
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeMap
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeQueue
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeStack
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeBlockQueue
OpenHarmony C++公共基礎(chǔ)類庫應(yīng)用案例:Thread
OpenHarmony C++公共基礎(chǔ)類庫應(yīng)用案例:Thread
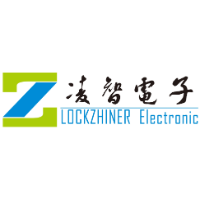
OpenHarmony C++公共基礎(chǔ)類庫應(yīng)用案例:HelloWorld
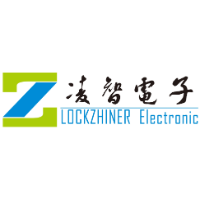
OpenHarmony標(biāo)準(zhǔn)系統(tǒng)C++公共基礎(chǔ)類庫案例:HelloWorld
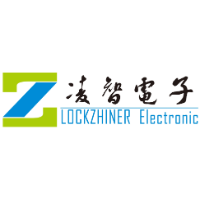
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeBlockQueue
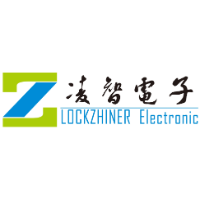
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeStack
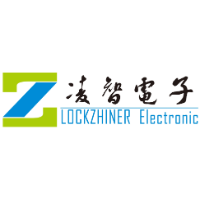
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeQueue
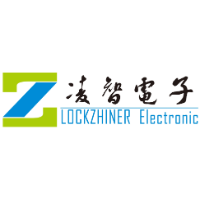
基于OpenHarmony標(biāo)準(zhǔn)系統(tǒng)的C++公共基礎(chǔ)類庫案例:SafeMap
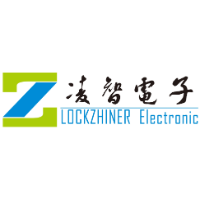
評(píng)論