本文詳細解析了 Spring 的內(nèi)置作用域,包括 Singleton、Prototype、Request、Session、Application 和 WebSocket 作用域,并通過實例講解了它們在實際開發(fā)中的應用。特別是 Singleton 和 Prototype 作用域,我們深入討論了它們的定義、用途以及如何處理相關(guān)的線程安全問題。通過閱讀本文,讀者可以更深入地理解 Spring 作用域,并在實際開發(fā)中更有效地使用
1. Spring 的內(nèi)置作用域
我們來看看 Spring 內(nèi)置的作用域類型。在 5.x 版本中,Spring 內(nèi)置了六種作用域:
singleton:在 IOC 容器中,對應的 Bean 只有一個實例,所有對它的引用都指向同一個對象。這種作用域非常適合對于無狀態(tài)的 Bean,比如工具類或服務類。
prototype:每次請求都會創(chuàng)建一個新的 Bean 實例,適合對于需要維護狀態(tài)的 Bean。
request:在 Web 應用中,為每個 HTTP 請求創(chuàng)建一個 Bean 實例。適合在一個請求中需要維護狀態(tài)的場景,如跟蹤用戶行為信息。
session:在 Web 應用中,為每個 HTTP 會話創(chuàng)建一個 Bean 實例。適合需要在多個請求之間維護狀態(tài)的場景,如用戶會話。
application:在整個 Web 應用期間,創(chuàng)建一個 Bean 實例。適合存儲全局的配置數(shù)據(jù)等。
websocket:在每個 WebSocket 會話中創(chuàng)建一個 Bean 實例。適合 WebSocket 通信場景。
我們需要重點學習兩種作用域:singleton 和 prototype。在大多數(shù)情況下 singleton 和 prototype 這兩種作用域已經(jīng)足夠滿足需求。
2. singleton 作用域
2.1 singleton 作用域的定義和用途
Singleton 是 Spring 的默認作用域。在這個作用域中,Spring 容器只會創(chuàng)建一個實例,所有對該 bean 的請求都將返回這個唯一的實例。 例如,我們定義一個名為 Plaything 的類,并將其作為一個 bean:
@Component public class Plaything { public Plaything() { System.out.println("Plaything constructor run ..."); } }
在這個例子中,Plaything 是一個 singleton 作用域的 bean。無論我們在應用中的哪個地方請求這個 bean,Spring 都會返回同一個 Plaything 實例。
下面的例子展示了如何創(chuàng)建一個單實例的 Bean:
package com.example.demo.bean; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Kid { private Plaything plaything; @Autowired public void setPlaything(Plaything plaything) { this.plaything = plaything; } public Plaything getPlaything() { return plaything; } } package com.example.demo.bean; import org.springframework.stereotype.Component; @Component public class Plaything { public Plaything() { System.out.println("Plaything constructor run ..."); } }
這里可以在 Plaything 類加上 @Scope (BeanDefinition.SCOPE_SINGLETON),但是因為是默認作用域是 Singleton,所以沒必要加。
package com.example.demo.configuration; import com.example.demo.bean.Kid; import com.example.demo.bean.Plaything; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; @Configuration public class BeanScopeConfiguration { @Bean public Kid kid1(Plaything plaything1) { Kid kid = new Kid(); kid.setPlaything(plaything1); return kid; } @Bean public Kid kid2(Plaything plaything2) { Kid kid = new Kid(); kid.setPlaything(plaything2); return kid; } } package com.example.demo.application; import com.example.demo.bean.Kid; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.context.ApplicationContext; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import org.springframework.context.annotation.ComponentScan; @SpringBootApplication @ComponentScan("com.example") public class DemoApplication { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(DemoApplication.class); context.getBeansOfType(Kid.class).forEach((name, kid) -> { System.out.println(name + " : " + kid.getPlaything()); }); } } 在 Spring IoC 容器的工作中,掃描過程只會創(chuàng)建 bean 的定義,真正的 bean 實例是在需要注入或者通過 getBean 方法獲取時才會創(chuàng)建。這個過程被稱為 bean 的初始化。
這里運行 ctx.getBeansOfType (Kid.class).forEach ((name, kid) -> System.out.println (name + ":" + kid.getPlaything ())); 時,Spring IoC 容器會查找所有的 Kid 類型的 bean 定義,然后為每一個找到的 bean 定義創(chuàng)建實例(如果這個 bean 定義還沒有對應的實例),并注入相應的依賴。
運行結(jié)果: 三個 Kid 的 Plaything bean 是相同的,說明默認情況下 Plaything 是一個單例 bean,整個 Spring 應用中只有一個 Plaything bean 被創(chuàng)建。 為什么會有 3 個 kid?
Kid: 這個是通過在 Kid 類上標注的 @Component 注解自動創(chuàng)建的。Spring 在掃描時發(fā)現(xiàn)這個注解,就會自動在 IOC 容器中注冊這個 bean。這個 Bean 的名字默認是將類名的首字母小寫 kid。
kid1: 在 BeanScopeConfiguration 中定義,通過 kid1 (Plaything plaything1) 方法創(chuàng)建,并且注入了 plaything1。
kid2: 在 BeanScopeConfiguration 中定義,通過 kid2 (Plaything plaything2) 方法創(chuàng)建,并且注入了 plaything2。
2.2 singleton 作用域線程安全問題
需要注意的是,雖然 singleton Bean 只會有一個實例,但 Spring 并不會解決其線程安全問題,開發(fā)者需要根據(jù)實際場景自行處理。 我們通過一個代碼示例來說明在多線程環(huán)境中出現(xiàn) singleton Bean 的線程安全問題。 首先,我們創(chuàng)建一個名為 Counter 的 singleton Bean,這個 Bean 有一個 count 變量,提供 increment 方法來增加 count 的值:
package com.example.demo.bean; import org.springframework.stereotype.Component; @Component public class Counter { private int count = 0; public int increment() { return ++count; } }
然后,我們創(chuàng)建一個名為 CounterService 的 singleton Bean,這個 Bean 依賴于 Counter,在 increaseCount 方法中,我們調(diào)用 counter.increment 方法:
package com.example.demo.service; import com.example.demo.bean.Counter; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; @Service public class CounterService { @Autowired private final Counter counter; public void increaseCount() { counter.increment(); } }我們在多線程環(huán)境中調(diào)用 counterService.increaseCount 方法時,就可能出現(xiàn)線程安全問題。因為 counter.increment 方法并非線程安全,多個線程同時調(diào)用此方法可能會導致 count 值出現(xiàn)預期外的結(jié)果。
要解決這個問題,我們需要使 counter.increment 方法線程安全。
這里可以使用原子變量,在 Counter 類中,我們可以使用 AtomicInteger 來代替 int 類型的 count,因為 AtomicInteger 類中的方法是線程安全的,且其性能通常優(yōu)于 synchronized 關(guān)鍵字。
?
package com.example.demo.bean; import org.springframework.stereotype.Component; import java.util.concurrent.atomic.AtomicInteger; @Component public class Counter { private AtomicInteger count = new AtomicInteger(0); public int increment() { return count.incrementAndGet(); } }盡管優(yōu)化后已經(jīng)使 Counter 類線程安全,但在設計 Bean 時,我們應該盡可能地減少可變狀態(tài)。這是因為可變狀態(tài)使得并發(fā)編程變得復雜,而無狀態(tài)的 Bean 通常更容易理解和測試。 什么是無狀態(tài)的 Bean 呢??如果一個 Bean 不持有任何狀態(tài)信息,也就是說,同樣的輸入總是會得到同樣的輸出,那么這個 Bean 就是無狀態(tài)的。反之,則是有狀態(tài)的 Bean。
?
3. prototype 作用域
3.1 prototype 作用域的定義和用途
在 prototype 作用域中,Spring 容器會為每個請求創(chuàng)建一個新的 bean 實例。 例如,我們定義一個名為 Plaything 的類,并將其作用域設置為 prototype:
?
package com.example.demo.bean; import org.springframework.beans.factory.config.BeanDefinition; import org.springframework.context.annotation.Scope; import org.springframework.stereotype.Component; @Component @Scope(BeanDefinition.SCOPE_PROTOTYPE) public class Plaything { public Plaything() { System.out.println("Plaything constructor run ..."); } }在這個例子中,Plaything 是一個 prototype 作用域的 bean。每次我們請求這個 bean,Spring 都會創(chuàng)建一個新的 Plaything 實例。 我們只需要修改上面的 Plaything 類,其他的類不用動。 打印結(jié)果:
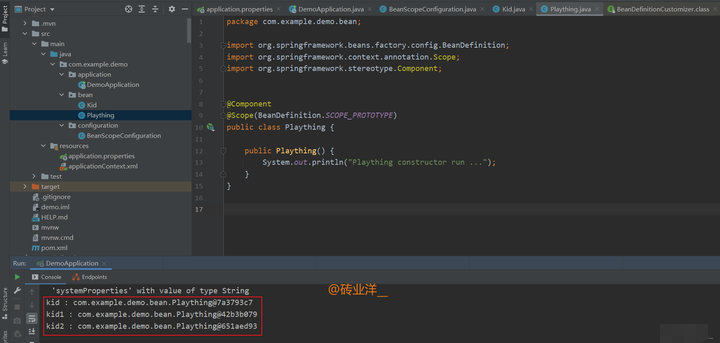
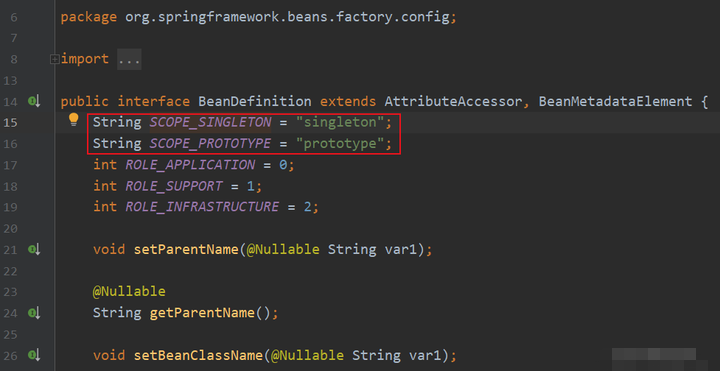
?
3.2 prototype 作用域在開發(fā)中的例子
以我個人來說,我在 excel 多線程上傳的時候用到過這個,當時是 EasyExcel 框架,我給一部分關(guān)鍵代碼展示一下如何在 Spring 中使用 prototype 作用域來處理多線程環(huán)境下的任務(實際業(yè)務會更復雜),大家可以對比,如果用 prototype 作用域和使用 new 對象的形式在實際開發(fā)中有什么區(qū)別。 使用 prototype 作用域的例子
?
@Resource private ApplicationContext context; @PostMapping("/user/upload") public ResultModel upload(@RequestParam("multipartFile") MultipartFile multipartFile) { ...... ExecutorService es = new ThreadPoolExceutor(10, 16, 0L, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<>(2000), new ThreadPoolExecutor.CallerRunPolicy()); ...... EasyExcel.read(multipartFile.getInputStream(), UserDataUploadVO.class, new PageReadListener(dataList ->{ ...... // 多線程處理上傳excel數(shù)據(jù) Future> future = es.submit(context.getBean(AsyncUploadHandler.class, user, dataList, errorCount)); ...... })).sheet().doRead(); ...... }
?
AsyncUploadHandler.java
?
@Component @Scope(BeanDefinition.SCOPE_PROTOTYPE) public class AsyncUploadHandler implements Runnable { private User user; private ListAsyncUploadHandler 類是一個 prototype 作用域的 bean,它被用來處理上傳的 Excel 數(shù)據(jù)。由于并發(fā)上傳的每個任務可能需要處理不同的數(shù)據(jù),并且可能需要在不同的用戶上下文中執(zhí)行,因此每個任務都需要有自己的 AsyncUploadHandler bean。這就是為什么需要將 AsyncUploadHandler 定義為 prototype 作用域的原因。 由于 AsyncUploadHandler 是由 Spring 管理的,我們可以直接使用 @Resource 注解來注入其他的 bean,例如 RedisService 和 CompanyManagementMapper。 把 AsyncUploadHandler 交給 Spring 容器管理,里面依賴的容器對象可以直接用 @Resource 注解注入。如果采用 new 出來的對象,那么這些對象只能從外面注入好了再傳入進去。 不使用 prototype 作用域改用 new 對象的例子dataList; private AtomicInteger errorCount; @Resource private RedisService redisService; ...... @Resource private CompanyManagementMapper companyManagementMapper; public AsyncUploadHandler(user, List dataList, AtomicInteger errorCount) { this.user = user; this.dataList = dataList; this.errorCount = errorCount; } @Override public void run() { ...... } ...... }
@PostMapping("/user/upload") public ResultModel upload(@RequestParam("multipartFile") MultipartFile multipartFile) { ...... ExecutorService es = new ThreadPoolExceutor(10, 16, 0L, TimeUnit.MILLISECONDS, new LinkedBlockingQueue<>(2000), new ThreadPoolExecutor.CallerRunPolicy()); ...... EasyExcel.read(multipartFile.getInputStream(), UserDataUploadVO.class, new PageReadListener(dataList ->{ ...... // 多線程處理上傳excel數(shù)據(jù) Future> future = es.submit(new AsyncUploadHandler(user, dataList, errorCount, redisService, companyManagementMapper)); ...... })).sheet().doRead(); ...... }
AsyncUploadHandler.java
public class AsyncUploadHandler implements Runnable { private User user; private List如果直接新建 AsyncUploadHandler 對象,則需要手動傳入所有的依賴,這會使代碼變得更復雜更難以管理,而且還需要手動管理 AsyncUploadHandler 的生命周期。dataList; private AtomicInteger errorCount; private RedisService redisService; private CompanyManagementMapper companyManagementMapper; ...... public AsyncUploadHandler(user, List dataList, AtomicInteger errorCount, RedisService redisService, CompanyManagementMapper companyManagementMapper) { this.user = user; this.dataList = dataList; this.errorCount = errorCount; this.redisService = redisService; this.companyManagementMapper = companyManagementMapper; } @Override public void run() { ...... } ...... }
4. request 作用域(了解)
request 作用域:Bean 在一個 HTTP 請求內(nèi)有效。當請求開始時,Spring 容器會為每個新的 HTTP 請求創(chuàng)建一個新的 Bean 實例,這個 Bean 在當前 HTTP 請求內(nèi)是有效的,請求結(jié)束后,Bean 就會被銷毀。如果在同一個請求中多次獲取該 Bean,就會得到同一個實例,但是在不同的請求中獲取的實例將會不同。
?
@Component @Scope(value = WebApplicationContext.SCOPE_REQUEST, proxyMode = ScopedProxyMode.TARGET_CLASS) public class RequestScopedBean { // 在一次Http請求內(nèi)共享的數(shù)據(jù) private String requestData; public void setRequestData(String requestData) { this.requestData = requestData; } public String getRequestData() { return this.requestData; } }上述 Bean 在一個 HTTP 請求的生命周期內(nèi)是一個單例,每個新的 HTTP 請求都會創(chuàng)建一個新的 Bean 實例。
5. session 作用域(了解)
session 作用域:Bean 是在同一個 HTTP 會話(Session)中是單例的。也就是說,從用戶登錄開始,到用戶退出登錄(或者 Session 超時)結(jié)束,這個過程中,不管用戶進行了多少次 HTTP 請求,只要是在同一個會話中,都會使用同一個 Bean 實例。
@Component @Scope(value = WebApplicationContext.SCOPE_SESSION, proxyMode = ScopedProxyMode.TARGET_CLASS) public class SessionScopedBean { // 在一個Http會話內(nèi)共享的數(shù)據(jù) private String sessionData; public void setSessionData(String sessionData) { this.sessionData = sessionData; } public String getSessionData() { return this.sessionData; } }這樣的設計對于存儲和管理會話級別的數(shù)據(jù)非常有用,例如用戶的登錄信息、購物車信息等。因為它們是在同一個會話中保持一致的,所以使用 session 作用域的 Bean 可以很好地解決這個問題。 但是實際開發(fā)中沒人這么干,會話 id 都會存在數(shù)據(jù)庫,根據(jù)會話 id 就能在各種表中獲取數(shù)據(jù),避免頻繁查庫也是把關(guān)鍵信息序列化后存在 Redis。
?
6. application 作用域(了解)
application 作用域:在整個 Web 應用的生命周期內(nèi),Spring 容器只會創(chuàng)建一個 Bean 實例。這個 Bean 在 Web 應用的生命周期內(nèi)都是有效的,當 Web 應用停止后,Bean 就會被銷毀。
?
@Component @Scope(value = WebApplicationContext.SCOPE_APPLICATION, proxyMode = ScopedProxyMode.TARGET_CLASS) public class ApplicationScopedBean { // 在整個Web應用的生命周期內(nèi)共享的數(shù)據(jù) private String applicationData; public void setApplicationData(String applicationData) { this.applicationData = applicationData; } public String getApplicationData() { return this.applicationData; } }如果在一個 application 作用域的 Bean 上調(diào)用 setter 方法,那么這個變更將對所有用戶和會話可見。后續(xù)對這個 Bean 的所有調(diào)用(包括 getter 和 setter)都將影響到同一個 Bean 實例,后面的調(diào)用會覆蓋前面的狀態(tài)。
7. websocket 作用域(了解)
websocket 作用域:Bean 在每一個新的 WebSocket 會話中都會被創(chuàng)建一次,就像 session 作用域的 Bean 在每一個 HTTP 會話中都會被創(chuàng)建一次一樣。這個 Bean 在整個 WebSocket 會話內(nèi)都是有效的,當 WebSocket 會話結(jié)束后,Bean 就會被銷毀。
@Component @Scope(value = "websocket", proxyMode = ScopedProxyMode.TARGET_CLASS) public class WebSocketScopedBean { // 在一個WebSocket會話內(nèi)共享的數(shù)據(jù) private String socketData; public void setSocketData(String socketData) { this.socketData = socketData; } public String getSocketData() { return this.socketData; } }上述 Bean 在一個 WebSocket 會話的生命周期內(nèi)是一個單例,每個新的 WebSocket 會話都會創(chuàng)建一個新的 Bean 實例。 這個作用域需要 Spring Websocket 模塊支持,并且應用需要配置為使用 websocket。
編輯:黃飛
?
?
?
?
評論